基于char* 设计一个字符串类MyString,具有构造函数、析构函数、复制构造函数。具体如下: MyString(); MyString(const char* cString); char at(int index) const; int length() const; void clear(); bool empty() const; int compare(const MyString& s) const; int compare(int index, int n, const MyString& s) const; void copy(char s[], int index , int n); char* data() const; int find(char ch) const ; int find(char ch ,int index) const; int find(const MyString& s, int index) const; 完整代码
时间: 2023-12-12 13:06:02 浏览: 111
以下是基于char*设计的字符串类MyString的完整代码:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class MyString {
private:
char* str; // 存储字符串的char指针
int len; // 字符串长度
public:
// 默认构造函数
MyString();
// 构造函数
MyString(const char* cString);
// 复制构造函数
MyString(const MyString& s);
// 析构函数
~MyString();
// 返回指定位置的字符
char at(int index) const;
// 返回字符串长度
int length() const;
// 清空字符串
void clear();
// 判断字符串是否为空
bool empty() const;
// 比较两个字符串的大小
int compare(const MyString& s) const;
// 比较指定位置开始的n个字符与另一个字符串的大小
int compare(int index, int n, const MyString& s) const;
// 复制指定长度的字符到另一个字符数组中
void copy(char s[], int index, int n);
// 返回存储字符串的char指针
char* data() const;
// 查找指定字符的位置
int find(char ch) const;
// 从指定位置开始查找指定字符的位置
int find(char ch, int index) const;
// 从指定位置开始查找另一个字符串的位置
int find(const MyString& s, int index) const;
};
// 默认构造函数
MyString::MyString() {
str = new char[1];
str[0] = '\0';
len = 0;
}
// 构造函数
MyString::MyString(const char* cString) {
len = strlen(cString);
str = new char[len + 1];
strcpy(str, cString);
}
// 复制构造函数
MyString::MyString(const MyString& s) {
len = s.len;
str = new char[len + 1];
strcpy(str, s.str);
}
// 析构函数
MyString::~MyString() {
delete[] str;
}
// 返回指定位置的字符
char MyString::at(int index) const {
if (index < 0 || index >= len) {
cerr << "Index out of range!" << endl;
exit(1);
}
return str[index];
}
// 返回字符串长度
int MyString::length() const {
return len;
}
// 清空字符串
void MyString::clear() {
str[0] = '\0';
len = 0;
}
// 判断字符串是否为空
bool MyString::empty() const {
return len == 0;
}
// 比较两个字符串的大小
int MyString::compare(const MyString& s) const {
return strcmp(str, s.str);
}
// 比较指定位置开始的n个字符与另一个字符串的大小
int MyString::compare(int index, int n, const MyString& s) const {
if (index < 0 || index > len || n < 0) {
cerr << "Invalid arguments!" << endl;
exit(1);
}
return strncmp(str + index, s.str, n);
}
// 复制指定长度的字符到另一个字符数组中
void MyString::copy(char s[], int index, int n) {
if (index < 0 || index > len || n < 0) {
cerr << "Invalid arguments!" << endl;
exit(1);
}
strncpy(s, str + index, n);
}
// 返回存储字符串的char指针
char* MyString::data() const {
return str;
}
// 查找指定字符的位置
int MyString::find(char ch) const {
char* p = strchr(str, ch);
if (p == NULL)
return -1;
else
return p - str;
}
// 从指定位置开始查找指定字符的位置
int MyString::find(char ch, int index) const {
if (index < 0 || index >= len) {
cerr << "Index out of range!" << endl;
exit(1);
}
char* p = strchr(str + index, ch);
if (p == NULL)
return -1;
else
return p - str;
}
// 从指定位置开始查找另一个字符串的位置
int MyString::find(const MyString& s, int index) const {
if (index < 0 || index >= len) {
cerr << "Index out of range!" << endl;
exit(1);
}
char* p = strstr(str + index, s.str);
if (p == NULL)
return -1;
else
return p - str;
}
// 测试代码
int main() {
MyString s1; // 默认构造函数
MyString s2("hello"); // 构造函数
MyString s3(s2); // 复制构造函数
cout << s1.length() << endl; // 0
cout << s2.length() << endl; // 5
cout << s3.length() << endl; // 5
s2.clear();
cout << s2.empty() << endl; // 1
cout << s3.compare(s2) << endl; // 1 (s3 > s2)
cout << s3.compare(1, 3, s2) << endl; // 1 ("ell" > "")
char s[10];
s3.copy(s, 1, 3);
s[3] = '\0';
cout << s << endl; // "ell"
cout << s3.data() << endl; // "hello"
cout << s3.find('l') << endl; // 2
cout << s3.find('l', 3) << endl; // 3
MyString s4("l");
cout << s3.find(s4, 2) << endl; // 2
return 0;
}
```
阅读全文
相关推荐
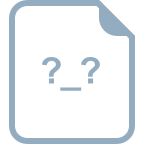














