基于char*设计一个字符串类Mystring
时间: 2023-06-12 17:04:20 浏览: 120
好的,下面是一个简单的 Mystring 类的设计:
```cpp
class Mystring {
private:
char* str;
int len;
public:
Mystring() : str(nullptr), len(0) {}
Mystring(const char* s) : str(nullptr), len(0) {
if (s) {
len = strlen(s);
str = new char[len + 1];
strcpy(str, s);
}
}
Mystring(const Mystring& rhs) : str(nullptr), len(rhs.len) {
if (rhs.str) {
str = new char[len + 1];
strcpy(str, rhs.str);
}
}
~Mystring() {
delete[] str;
}
Mystring& operator=(const Mystring& rhs) {
if (this != &rhs) {
delete[] str;
len = rhs.len;
if (rhs.str) {
str = new char[len + 1];
strcpy(str, rhs.str);
} else {
str = nullptr;
}
}
return *this;
}
Mystring& operator+=(const Mystring& rhs) {
int newlen = len + rhs.len;
char* newstr = new char[newlen + 1];
if (str) {
strcpy(newstr, str);
delete[] str;
}
strcpy(newstr + len, rhs.str);
str = newstr;
len = newlen;
return *this;
}
friend Mystring operator+(Mystring lhs, const Mystring& rhs) {
lhs += rhs;
return lhs;
}
char operator[](int index) const {
if (index < 0 || index >= len) {
throw std::out_of_range("Mystring out of range");
}
return str[index];
}
char& operator[](int index) {
if (index < 0 || index >= len) {
throw std::out_of_range("Mystring out of range");
}
return str[index];
}
const char* c_str() const {
return str ? str : "";
}
int length() const {
return len;
}
// 可以根据需要添加其他函数或运算符重载
};
```
这个类包含了一些常用的字符串操作,例如构造函数、拷贝构造函数、析构函数、赋值运算符、加法运算符、下标运算符、c_str() 函数和 length() 函数等等。其中,c_str() 函数返回一个指向字符串的指针,length() 函数返回字符串的长度。需要注意的是,这个类中使用了动态内存分配,因此需要特别注意内存泄漏和内存安全问题。
阅读全文
相关推荐














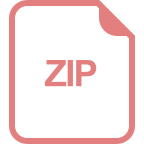