.请设计一个字符串类Mystring, 通过该类实现与字符串相关的各种操作。 字符串类的主要功能包括:字符串长度计算、 字符串拼接、字符串大小比较、字符串元素查找和替换操作、对象空间释放。要求使用字符数组存储字符串,不允许使用string类型.用c++
时间: 2024-12-13 17:27:50 浏览: 6
在 C++ 中,我们可以创建一个名为 `MyString` 的自定义字符串类,它使用字符数组作为底层数据结构,以实现上述的各种操作。以下是这个类的基本设计:
```cpp
#include <iostream>
class MyString {
private:
char* str; // 存储字符串的字符数组
int length; // 字符串长度
public:
// 构造函数
MyString(const char* initStr = "") {
if (initStr) {
length = strlen(initStr);
str = new char[length + 1]; // 为null字符留位置
std::strcpy(str, initStr);
} else {
length = 0;
str = new char[1];
str[0] = '\0';
}
}
// 获取字符串长度
int getLength() const {
return length;
}
// 拼接字符串
void concat(const MyString& other) {
int combinedLength = this->length + other.getLength();
char* combined = new char[combinedLength + 1];
std::strncpy(combined, this->str, this->length);
combined[this->length] = '\0';
std::strcat(combined, other.str);
delete[] this->str;
this->str = combined;
this->length = combinedLength;
}
// 比较字符串大小
bool compare(const MyString& other) const {
if (this->length != other.length)
return this->length < other.length;
for (int i = 0; i < this->length; ++i) {
if (this->str[i] < other.str[i])
return true;
if (this->str[i] > other.str[i])
return false;
}
return false; // 如果所有字符都相等,返回false
}
// 查找字符
int find(char target) const {
for (int i = 0; i < this->length; ++i) {
if (this->str[i] == target)
return i;
}
return -1; // 如果找不到,返回-1
}
// 替换字符
void replace(char oldChar, char newChar) {
for (int i = 0; i < this->length; ++i) {
if (this->str[i] == oldChar) {
this->str[i] = newChar;
}
}
}
// 释放内存
~MyString() {
delete[] str;
}
};
// 使用示例
int main() {
MyString s1("Hello");
MyString s2("World");
s1.concat(s2);
std::cout << "Concatenated: " << s1.getString() << std::endl;
if (s1.compare("World")) {
std::cout << "s1 is smaller than World" << std::endl;
} else {
std::cout << "s1 is equal to or greater than World" << std::endl;
}
int index = s1.find('o');
if (index != -1)
std::cout << "Found 'o' at position " << index << std::endl;
s1.replace('W', 'X');
std::cout << "After replacing: " << s1.getString() << std::endl;
return 0;
}
```
阅读全文
相关推荐
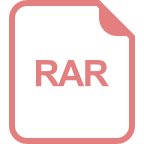
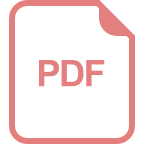
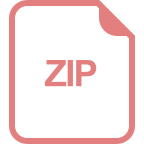








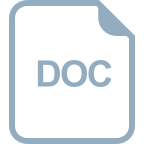
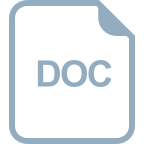





