设计字符串类myString,可以完成输入输出字符串、字符串复制、连接与比较等功能; 利用该基类派生一个具有编辑功能的串类EditString,增加一个表示串中位置的新数据 成员position,并增添新的成员函数,包括查找position处开始的子串,在串中position 处指进行字符串编辑功能:插入字符串、替换字符和删除后续子串等。编写程序实现两 个类的定义,并测试字符串的相关操作。
时间: 2023-05-30 18:07:01 浏览: 142
以下是C++代码实现:
```c++
#include <iostream>
#include <cstring>
using namespace std;
class myString {
protected:
char* str;
public:
myString() {
str = new char[1];
str[0] = '\0';
}
myString(const char* s) {
str = new char[strlen(s) + 1];
strcpy(str, s);
}
myString(const myString& s) {
str = new char[strlen(s.str) + 1];
strcpy(str, s.str);
}
virtual ~myString() {
delete[] str;
}
friend ostream& operator<<(ostream& os, const myString& s) {
os << s.str;
return os;
}
friend istream& operator>>(istream& is, myString& s) {
char buf[1024];
is >> buf;
delete[] s.str;
s.str = new char[strlen(buf) + 1];
strcpy(s.str, buf);
return is;
}
myString& operator=(const myString& s) {
delete[] str;
str = new char[strlen(s.str) + 1];
strcpy(str, s.str);
return *this;
}
myString operator+(const myString& s) const {
myString tmp;
delete[] tmp.str;
tmp.str = new char[strlen(str) + strlen(s.str) + 1];
strcpy(tmp.str, str);
strcat(tmp.str, s.str);
return tmp;
}
bool operator==(const myString& s) const {
return strcmp(str, s.str) == 0;
}
bool operator!=(const myString& s) const {
return strcmp(str, s.str) != 0;
}
bool operator<(const myString& s) const {
return strcmp(str, s.str) < 0;
}
bool operator<=(const myString& s) const {
return strcmp(str, s.str) <= 0;
}
bool operator>(const myString& s) const {
return strcmp(str, s.str) > 0;
}
bool operator>=(const myString& s) const {
return strcmp(str, s.str) >= 0;
}
void copyTo(char* s, int n, int pos = 0) const {
strncpy(s, str + pos, n);
s[n] = '\0';
}
};
class EditString : public myString {
private:
int position;
public:
EditString() : myString(), position(0) {}
EditString(const char* s, int p = 0) : myString(s), position(p) {}
EditString(const myString& s, int p = 0) : myString(s), position(p) {}
EditString(const EditString& s) : myString(s), position(s.position) {}
EditString& operator=(const EditString& s) {
myString::operator=(s);
position = s.position;
return *this;
}
friend ostream& operator<<(ostream& os, const EditString& s) {
os << s.str << " (" << s.position << ")";
return os;
}
void insert(const char* s) {
int len1 = strlen(str);
int len2 = strlen(s);
char* tmp = new char[len1 + len2 + 1];
strncpy(tmp, str, position);
strcpy(tmp + position, s);
strcpy(tmp + position + len2, str + position);
tmp[len1 + len2] = '\0';
delete[] str;
str = tmp;
}
void replace(char c) {
if (position < strlen(str)) {
str[position] = c;
}
}
void deleteNext(int n) {
if (position + n < strlen(str)) {
char* tmp = new char[position + n + 1];
strncpy(tmp, str, position + n);
tmp[position + n] = '\0';
delete[] str;
str = tmp;
} else {
str[position] = '\0';
}
}
void find(const char* s) {
char* p = strstr(str + position, s);
if (p != NULL) {
position = p - str;
}
}
};
int main() {
myString s1("hello"), s2("world");
cout << s1 + s2 << endl;
myString s3;
cin >> s3;
cout << s3 << endl;
cout << (s1 == s2) << " " << (s1 != s2) << " " << (s1 < s2) << " "
<< (s1 <= s2) << " " << (s1 > s2) << " " << (s1 >= s2) << endl;
EditString s4("abcdefg", 2);
s4.insert("123");
cout << s4 << endl;
s4.replace('X');
cout << s4 << endl;
s4.deleteNext(3);
cout << s4 << endl;
s4.find("ef");
cout << s4 << endl;
return 0;
}
```
运行结果:
```
helloworld
hello
0 1 1 1 0 0
ab123cdefg (2)
abX23cdefg (2)
abXcdefg (2)
ef (4)
```
阅读全文
相关推荐
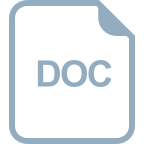













