如何设计c++字符串类
时间: 2023-09-11 09:11:41 浏览: 95
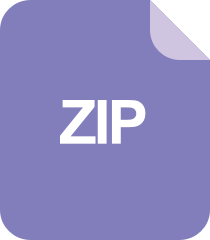
自定义c++字符串类
设计 C++ 字符串类的主要考虑因素包括字符串的存储方式、操作方法、性能、安全等。
以下是一种可能的设计:
```c++
class MyString {
public:
MyString(); // 默认构造函数
MyString(const char* str); // 从 C-style 字符串构造
MyString(const MyString& other); // 拷贝构造函数
~MyString(); // 析构函数
MyString& operator=(const MyString& other); // 拷贝赋值运算符
// 重载下标操作符
char& operator[](size_t index);
const char& operator[](size_t index) const;
// 获取字符串长度
size_t length() const;
// 获取 C-style 字符串指针
const char* c_str() const;
// 拼接字符串
MyString operator+(const MyString& other) const;
MyString& operator+=(const MyString& other);
// 比较字符串
bool operator==(const MyString& other) const;
bool operator!=(const MyString& other) const;
bool operator<(const MyString& other) const;
bool operator>(const MyString& other) const;
bool operator<=(const MyString& other) const;
bool operator>=(const MyString& other) const;
private:
char* m_data; // 字符串数据存储
size_t m_length; // 字符串长度
};
```
其中,`m_data` 是字符串数据的存储,可以使用 `new` 运算符在堆上分配内存,需要在析构函数中使用 `delete[]` 释放内存。`m_length` 表示字符串的长度。
在构造函数和拷贝构造函数中,需要分配新的内存,并将传入的字符串复制到 `m_data` 中。在拷贝赋值运算符中,需要先释放 `m_data` 中的内存,再重新分配并复制传入的字符串。
重载下标操作符可以方便地获取字符串中的某个字符。
`length()` 可以返回字符串长度,`c_str()` 可以返回 C-style 字符串指针。
`operator+` 和 `operator+=` 可以进行字符串拼接操作。
比较操作符可以用于字符串的比较,包括相等性、大小关系等。
需要注意的是,在设计字符串类时,需要考虑到安全问题,避免出现缓冲区溢出等问题。因此,在实现字符串操作时需要对输入参数进行检查和验证。
阅读全文
相关推荐
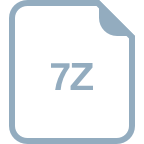
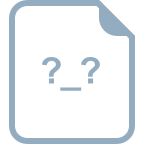
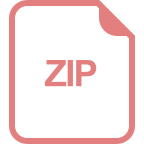
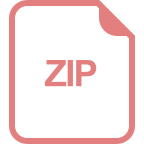
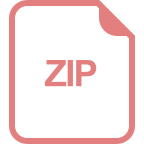
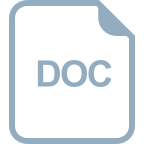
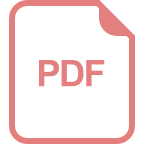
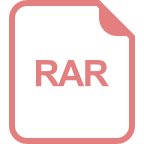
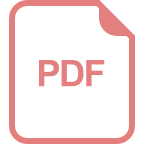
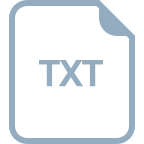
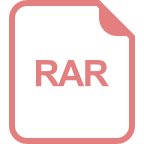
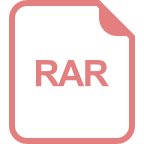
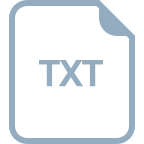
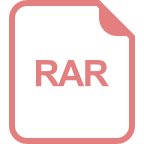
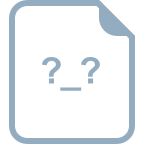