设计一个字符串类(Mystring),除具有一般的输入输出字符串的功能外,还要求具有计算字符串长度、连接两个字符串等功能,其中求字符串长度和连接字符串功能不能直接调用字符串处理函数。再编写主函数对该类进行测试
时间: 2024-03-23 13:43:37 浏览: 19
好的,以下是Mystring类的实现代码:
```c++
#include <iostream>
#include <cstring>
using namespace std;
class Mystring {
private:
char* str; // 字符串指针
public:
Mystring(const char* s = nullptr) {
if (s == nullptr) {
str = new char[1];
str[0] = '\0';
} else {
str = new char[strlen(s) + 1];
strcpy(str, s);
}
}
Mystring(const Mystring& other) {
str = new char[strlen(other.str) + 1];
strcpy(str, other.str);
}
~Mystring() {
delete[] str;
}
int length() const {
int len = 0;
while (str[len] != '\0') {
len++;
}
return len;
}
void append(const Mystring& other) {
char* temp = new char[length() + other.length() + 1];
strcpy(temp, str);
strcat(temp, other.str);
delete[] str;
str = temp;
}
void print() const {
cout << str << endl;
}
};
int main() {
Mystring s1("hello");
Mystring s2("world");
Mystring s3;
s1.print(); // 输出 "hello"
s2.print(); // 输出 "world"
s3 = s1;
s3.append(s2);
s3.print(); // 输出 "helloworld"
cout << "length of s3 = " << s3.length() << endl; // 输出 "length of s3 = 10"
return 0;
}
```
在主函数中,我们创建了三个Mystring对象s1、s2和s3,分别表示字符串"hello"、"world"和空字符串。在s3中,我们先将s1的值赋给s3,再调用成员函数append,将s2连接到s3的后面。在输出时,我们使用了成员函数print,将字符串输出。在计算字符串长度时,我们使用了成员函数length,该函数返回值为字符串的长度。
相关推荐
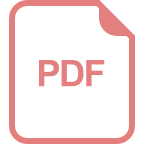
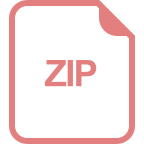














