完善自定义字符串类mystring,函数包括构造函数、复制构造函数、析构函数,并重载“[ ]”、”=” 、“+”(连接)、“<”、“>”、“==”(比较)。
时间: 2023-10-19 17:05:20 浏览: 68
好的,我理解了你的问题。以下是一个简单的实现,仅供参考:
```cpp
#include <iostream>
#include <cstring>
class mystring {
public:
mystring(const char* str = nullptr) {
if(str == nullptr) {
m_data = new char[1];
m_data[0] = '\0';
} else {
int len = strlen(str);
m_data = new char[len + 1];
strcpy(m_data, str);
}
}
mystring(const mystring& other) {
int len = strlen(other.m_data);
m_data = new char[len + 1];
strcpy(m_data, other.m_data);
}
~mystring() {
delete[] m_data;
}
mystring& operator=(const mystring& other) {
if(this == &other) {
return *this;
}
delete[] m_data;
int len = strlen(other.m_data);
m_data = new char[len + 1];
strcpy(m_data, other.m_data);
return *this;
}
mystring operator+(const mystring& other) {
mystring new_str;
int len = strlen(m_data) + strlen(other.m_data);
new_str.m_data = new char[len + 1];
strcpy(new_str.m_data, m_data);
strcat(new_str.m_data, other.m_data);
return new_str;
}
char& operator[](int index) {
return m_data[index];
}
bool operator<(const mystring& other) {
return strcmp(m_data, other.m_data) < 0;
}
bool operator>(const mystring& other) {
return strcmp(m_data, other.m_data) > 0;
}
bool operator==(const mystring& other) {
return strcmp(m_data, other.m_data) == 0;
}
private:
char* m_data;
};
int main() {
mystring str1("Hello");
mystring str2("World");
mystring str3 = str1 + str2;
std::cout << str3[0] << std::endl;
std::cout << (str1 < str2) << std::endl;
std::cout << (str1 > str2) << std::endl;
std::cout << (str1 == str2) << std::endl;
std::cout << (str1 == mystring("Hello")) << std::endl;
return 0;
}
```
在这个实现中,我们首先定义了一个字符串类 `mystring`,包括构造函数、复制构造函数、析构函数,并重载了 `[]`、`=`、`+`、`<`、`>`、`==` 等操作符。
在构造函数中,我们根据传入的字符串长度动态分配内存,并将字符串内容复制到类中的成员变量中。在复制构造函数和析构函数中,我们分别实现了深拷贝和释放内存的操作。
在 `=` 操作符中,我们首先判断是否为自己赋值,如果是则直接返回,否则先释放原有内存,再根据传入的字符串长度动态分配内存,并将字符串内容复制到类中的成员变量中。
在 `+` 操作符中,我们首先计算出两个字符串的长度,然后动态分配内存,将两个字符串连接起来,最后返回一个新的字符串。
在 `[]` 操作符中,我们直接返回类中成员变量中对应的字符。
在 `<`、`>`、`==` 操作符中,我们使用 `strcmp` 函数进行字符串比较,并返回对应的比较结果。
在 `main` 函数中,我们测试了字符串的各种操作,包括索引、比较、连接等。
阅读全文
相关推荐
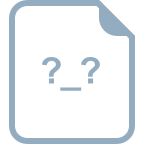




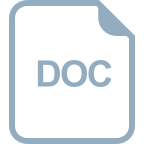









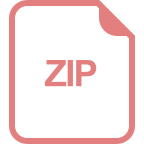