如何把一个文件复制到另一个文件C语言
时间: 2023-07-04 19:11:31 浏览: 85
您可以使用C语言的标准库函数 `fopen()`、`fread()` 和 `fwrite()` 来实现文件的复制。具体步骤如下:
1. 打开要复制的源文件和目标文件,使用 `fopen()` 函数分别打开这两个文件,打开方式为二进制读取和二进制写入模式,如下所示:
```c
FILE *source_file, *target_file;
source_file = fopen("source_file_path", "rb");
target_file = fopen("target_file_path", "wb");
```
2. 从源文件中读取数据,使用 `fread()` 函数从源文件中读取指定长度的数据,如下所示:
```c
char buffer[1024];
size_t bytes_read;
while ((bytes_read = fread(buffer, 1, sizeof(buffer), source_file)) > 0) {
fwrite(buffer, 1, bytes_read, target_file);
}
```
3. 关闭文件,使用 `fclose()` 函数关闭源文件和目标文件,如下所示:
```c
fclose(source_file);
fclose(target_file);
```
完整的代码示例如下:
```c
#include <stdio.h>
int main() {
FILE *source_file, *target_file;
char buffer[1024];
size_t bytes_read;
// 打开源文件和目标文件
source_file = fopen("source_file_path", "rb");
target_file = fopen("target_file_path", "wb");
// 从源文件中读取数据,并写入到目标文件中
while ((bytes_read = fread(buffer, 1, sizeof(buffer), source_file)) > 0) {
fwrite(buffer, 1, bytes_read, target_file);
}
// 关闭文件
fclose(source_file);
fclose(target_file);
return 0;
}
```
请将代码中的 "source_file_path" 和 "target_file_path" 替换为实际的源文件和目标文件路径。
相关推荐
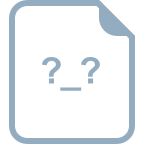
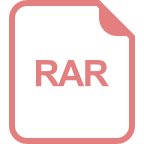














