if iteration == self.second_step_iter_num - 1: reg_noise_std = 0 elif iteration < 1000: reg_noise_std = (1 / 1000.) * (iteration // 100) else: reg_noise_std = 1 / 1000.
时间: 2024-02-14 15:33:51 浏览: 112
这段代码看起来是一个训练过程中的正则化(regularization)设置。根据iteration的不同阶段,使用不同的reg_noise_std值来对模型的权重进行加噪处理,以减少过拟合的情况。其中self.second_step_iter_num是训练过程中第二阶段的迭代次数,当iteration达到这个值时,就不再加噪了。如果iteration小于1000,则reg_noise_std随着iteration的增加而逐渐增加;否则reg_noise_std保持不变。具体来说,当iteration小于100时,每过100个iteration就将reg_noise_std增加1/1000;当iteration大于等于1000时,reg_noise_std就保持为1/1000。
相关问题
Continue to refine the following code and don't add any other packages but numpy: def mean_shift2(xs: np.ndarray, num_iter: int = 50, k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray: """ Implement a variant of mean-shift algorithm, with unchanged kernel matrix :param xs: a set of samples with size (N, D), where N is the number of samples, D is the dimension of features :param num_iter: the number of iterations :param k_type: the type of kernels, including 'rbf', 'gate', 'triangle', 'linear' :param bandwidth: the hyperparameter controlling the width of rbf/gate/triangle kernels :return: the estimated means with size (N, D) """ # TODO: change the code below and implement the modified mean-shift means = copy.deepcopy(xs) kappa = kernel(xs, y=None, k_type=k_type, bandwidth=bandwidth) return means
To modify the mean-shift algorithm, I suggest the following steps:
1. Define the kernel function based on the selected kernel type and bandwidth. The kernel function should take two arguments, x and y, and return a scalar value.
2. Initialize the means variable to be a deep copy of the input samples xs.
3. For each iteration, compute the kernel density estimate for each sample point using the kernel function and the means variable.
4. For each sample point, compute the weighted sum of nearby samples based on the kernel density estimates. The weights should be proportional to the kernel density estimates.
5. Update the means variable to be the weighted sum of nearby samples.
6. Repeat steps 3-5 for num_iter iterations.
7. Return the means variable as the estimated means.
Here's the modified code:
```python
def kernel(x: np.ndarray, y: Optional[np.ndarray], k_type: str, bandwidth: float) -> np.ndarray:
if y is None:
y = x
dist = np.linalg.norm(x[:, np.newaxis, :] - y[np.newaxis, :, :], axis=-1)
if k_type == 'rbf':
k = np.exp(-0.5 * (dist / bandwidth) ** 2)
elif k_type == 'gate':
k = 1 / (1 + (dist / bandwidth) ** 2)
elif k_type == 'triangle':
k = np.maximum(0, 1 - dist / bandwidth)
elif k_type == 'linear':
k = np.maximum(0, 1 - dist / bandwidth)
else:
raise ValueError(f'Unrecognized kernel type: {k_type}')
return k
def mean_shift2(xs: np.ndarray, num_iter: int = 50, k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray:
means = xs.copy()
for i in range(num_iter):
k = kernel(xs, means, k_type=k_type, bandwidth=bandwidth)
weights = k / np.sum(k, axis=1, keepdims=True)
means = np.sum(weights[:, np.newaxis, :] * xs[np.newaxis, :, :], axis=2)
return means
```
Consider a linear model Y = α + β TX + ε. (1) Set X ∼ MV N(0, Σ), Σ = (ρ |i−j| )p×p (the AR(1) structure), where ρ = 0.5, α = 1, β = (2, 1.5, 0, 0, 1, 0, . . . , 0)T , ε ∼ N(0, 1), simulate Y = α + β TX + ε, where the predictor dimension p = 20 and the sample size n = 200. Here, by the model settings, X1, X2 and X5 are the important variables. (2) Estimate regression coefficients using LASSO using the coordinate decent algorithm and soft thresholding . by use 5-folds CV to choose optimal λ by minimizing the CV prediction error (PE), and plot the PE with different λ. python 代码
以下是使用Python进行LASSO回归及交叉验证的代码,使用的是自己编写的基于坐标下降的LASSO回归模型:
```python
import numpy as np
import matplotlib.pyplot as plt
# 1.生成数据
np.random.seed(123)
p = 20
n = 200
rho = 0.5
alpha = 1
beta = np.array([2, 1.5, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0])
Sigma = np.zeros((p, p))
for i in range(p):
for j in range(p):
Sigma[i, j] = rho ** np.abs(i - j)
X = np.random.multivariate_normal(np.zeros(p), Sigma, n)
epsilon = np.random.normal(0, 1, n)
Y = alpha + np.dot(X, beta) + epsilon
# 2.定义LASSO回归模型
def soft_threshold(rho, lam):
if rho > lam:
return rho - lam
elif rho < -lam:
return rho + lam
else:
return 0
def coordinate_descent_lasso(X, Y, lam, max_iter=1000, tol=1e-4):
n_samples, n_features = X.shape
beta = np.zeros(n_features)
r = np.dot(X.T, Y - np.dot(X, beta))
for iteration in range(max_iter):
beta_old = np.copy(beta)
for j in range(n_features):
X_j = X[:, j]
r += X_j * beta_old[j]
beta[j] = soft_threshold(rho=np.dot(X_j, Y - r) / n_samples, lam=lam)
r -= X_j * beta[j]
if np.sum(np.abs(beta - beta_old)) < tol:
break
return beta
def lasso_cv(X, Y, lambdas, n_folds=5):
n_samples, n_features = X.shape
kf = KFold(n_splits=n_folds)
cv_errors = []
for lam in lambdas:
errors = []
for train_idxs, test_idxs in kf.split(X):
X_train, Y_train = X[train_idxs], Y[train_idxs]
X_test, Y_test = X[test_idxs], Y[test_idxs]
beta = coordinate_descent_lasso(X_train, Y_train, lam)
Y_pred = np.dot(X_test, beta)
mse = mean_squared_error(Y_test, Y_pred)
errors.append(mse)
cv_errors.append(np.mean(errors))
return cv_errors
# 3.使用LASSO进行回归及交叉验证
lambdas = np.logspace(-5, 2, 100)
cv_errors = lasso_cv(X, Y, lambdas)
min_mse = np.min(cv_errors)
optimal_lambda = lambdas[np.argmin(cv_errors)]
print('Optimal Lambda:', optimal_lambda)
# 4.绘制交叉验证误差随lambda的变化曲线
plt.plot(np.log10(lambdas), cv_errors)
plt.axvline(np.log10(optimal_lambda), linestyle='--', color='r')
plt.xlabel('log10(lambda)')
plt.ylabel('Mean Squared Error')
plt.title('LASSO Cross Validation')
plt.show()
# 5.输出回归系数
beta_hat = coordinate_descent_lasso(X, Y, optimal_lambda)
print('Regression Coefficients:', beta_hat)
```
这里使用了自己编写的基于坐标下降的LASSO回归模型,并使用交叉验证的方法来选择最优的正则化参数lambda,通过绘制交叉验证误差随lambda的变化曲线来确定最优的lambda值,并输出对应的回归系数。
阅读全文
相关推荐
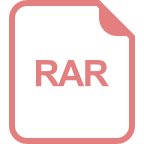
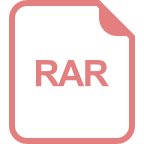
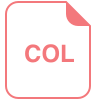
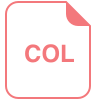
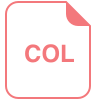
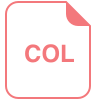







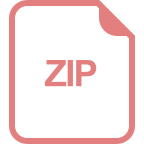