用C语言写下面的程序。将字符串str中的子字符串s1替换成新的子字符串s2(字符串长度<100),如果字符串str中有多个s1,则应全部替换,最后输出被替换后的str字符串。
时间: 2023-12-07 12:04:03 浏览: 90
以下是用C语言实现的程序:
```c
#include <stdio.h>
#include <string.h>
#define MAXLEN 100
void replace_str(char *str, char *s1, char *s2) {
int len1 = strlen(s1), len2 = strlen(s2);
char temp[MAXLEN + 1];
char *p;
while ((p = strstr(str, s1)) != NULL) {
strncpy(temp, str, p - str);
temp[p - str] = '\0';
strcat(temp, s2);
strcat(temp, p + len1);
strcpy(str, temp);
}
}
int main() {
char str[MAXLEN + 1], s1[MAXLEN + 1], s2[MAXLEN + 1];
printf("请输入原字符串:");
fgets(str, MAXLEN + 1, stdin);
printf("请输入要替换的字符串:");
fgets(s1, MAXLEN + 1, stdin);
printf("请输入新的字符串:");
fgets(s2, MAXLEN + 1, stdin);
// 去掉换行符
str[strlen(str) - 1] = '\0';
s1[strlen(s1) - 1] = '\0';
s2[strlen(s2) - 1] = '\0';
replace_str(str, s1, s2);
printf("替换后的字符串:%s\n", str);
return 0;
}
```
程序中使用了`strstr()`函数查找字符串中的子字符串,使用`strncpy()`和`strcat()`函数替换子字符串,最后输出替换后的字符串。注意要去掉每个输入字符串中的换行符。
阅读全文
相关推荐
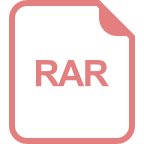












