qt中执行了stop函数后如何继续播放
时间: 2024-02-28 11:56:33 浏览: 26
在Qt中,如果您执行了QMediaPlayer的stop()函数,那么要继续播放,您可以调用QMediaPlayer的play()函数。以下是一个示例:
```
QMediaPlayer *player = new QMediaPlayer;
player->setMedia(QUrl::fromLocalFile("path/to/your/media/file"));
// 停止播放
player->stop();
// 继续播放
player->play();
```
请注意,如果您在执行stop()函数之前调用了pause()函数,则需要在调用play()函数之前先调用setMedia()函数来设置媒体文件。如果您只是在执行stop()函数之后想要继续播放,那么调用play()函数就足够了。
相关问题
Qt cpp 界面打开后 定时器延时0s执行doSomething函数
可以使用 Qt 中的 QTimer 类来实现定时器功能,具体实现步骤如下:
1. 在界面类的头文件中声明 QTimer 对象:`QTimer *timer;`
2. 在界面类的构造函数中创建 QTimer 对象,设置定时器的时间间隔,并将其与槽函数 `doSomething()` 关联:
```cpp
timer = new QTimer(this);
connect(timer, SIGNAL(timeout()), this, SLOT(doSomething()));
timer->setInterval(0); // 设置间隔为 0 毫秒
```
3. 在界面类的 `showEvent()` 函数中启动定时器:
```cpp
void MyWidget::showEvent(QShowEvent *event)
{
QWidget::showEvent(event);
timer->start();
}
```
这样,当界面显示出来时,定时器就会开始工作,等待 0 毫秒后就会触发 `doSomething()` 槽函数。注意,如果你想让 `doSomething()` 函数只执行一次,可以在槽函数中调用 `stop()` 函数停止定时器。
qt中qtimer定时器暂停后再次启动
在 Qt 中,可以通过调用 QTimer 类的 start() 函数来启动定时器,并通过调用 stop() 函数来停止定时器。如果需要暂停定时器后再次启动,可以先调用 stop() 函数停止定时器,然后记录定时器当前的时间间隔,并将定时器的计时器清零,最后再调用 start() 函数重新启动定时器。例如:
```
QTimer *timer = new QTimer(this);
connect(timer, SIGNAL(timeout()), this, SLOT(updateTime()));
timer->setInterval(1000); // 设置定时器的时间间隔为 1 秒
timer->start(); // 启动定时器
// 暂停定时器
timer->stop();
// 记录定时器当前的时间间隔,并将计时器清零
int remainingTime = timer->remainingTime();
timer->setInterval(remainingTime);
timer->setTimerType(Qt::PreciseTimer);
timer->setInterval(0);
// 再次启动定时器
timer->start();
```
在上面的例子中,首先通过 setTimerType() 函数将定时器的计时器类型设置为精确计时器,并通过 remainingTime() 函数获取定时器当前的剩余时间,然后将定时器的时间间隔设置为剩余时间,并将计时器清零,最后再次调用 start() 函数重新启动定时器。这样就可以实现暂停定时器后再次启动的功能了。
相关推荐
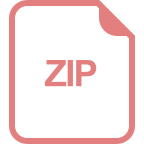
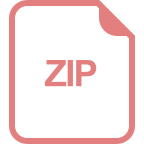
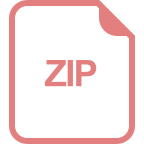












