用python 写一个基于数据库的出入库管理软件,软件有界面,有出库和入库设置,在右边有当前库存列表 程序并且功能完整点
时间: 2024-03-17 22:42:23 浏览: 20
好的,这是一个完整的基于数据库的出入库管理软件,包括GUI界面、数据库连接和操作、出入库设置和库存列表显示。
1. 确定数据库类型和创建数据库
与上面的步骤相同,您需要决定使用哪种数据库管理系统(DBMS),如MySQL或SQLite,并创建一个名为inventory的数据库。
2. 安装必要的库和框架
与上面的步骤相同,您需要安装必要的库和框架,包括pymysql、sqlite3、tkinter、Pillow和ttkthemes。
```python
import tkinter as tk
from tkinter import ttk
import pymysql
import sqlite3
from PIL import ImageTk, Image
from ttkthemes import ThemedTk
```
3. 创建Python文件并导入库和框架
与上面的步骤相同,您需要创建Python文件并导入所需的库和框架。
```python
root = ThemedTk(theme="arc")
root.title("出入库管理软件")
# 创建输入框和标签
item_label = ttk.Label(root, text="物品名称:")
item_label.grid(row=0, column=0, padx=5, pady=5, sticky="w")
item_entry = ttk.Entry(root, width=30)
item_entry.grid(row=0, column=1, padx=5, pady=5, sticky="w")
quantity_label = ttk.Label(root, text="数量:")
quantity_label.grid(row=1, column=0, padx=5, pady=5, sticky="w")
quantity_entry = ttk.Entry(root, width=10)
quantity_entry.grid(row=1, column=1, padx=5, pady=5, sticky="w")
# 创建按钮
add_button = ttk.Button(root, text="入库", command=add_item)
add_button.grid(row=0, column=2, padx=5, pady=5)
remove_button = ttk.Button(root, text="出库", command=remove_item)
remove_button.grid(row=1, column=2, padx=5, pady=5)
# 创建列表框
item_list = tk.Listbox(root, height=20, width=50)
item_list.grid(row=2, column=0, columnspan=3, padx=5, pady=5)
# 创建数据库连接
conn = pymysql.connect(host="localhost", user="root", password="password", db="inventory")
```
4. 编写数据库连接和操作代码
使用pymysql连接到MySQL数据库,并执行必要的查询和操作,例如添加物品、更新库存和获取库存列表。
```python
# 添加物品到数据库
def add_item():
item_name = item_entry.get()
quantity = int(quantity_entry.get())
cursor = conn.cursor()
cursor.execute("INSERT INTO items (name, quantity) VALUES (%s, %s)", (item_name, quantity))
conn.commit()
cursor.close()
update_list()
# 从数据库中删除物品
def remove_item():
selected_item = item_list.curselection()
if selected_item:
item_name = item_list.get(selected_item[0]).split(":")[0]
quantity = int(quantity_entry.get())
cursor = conn.cursor()
cursor.execute("UPDATE items SET quantity = quantity - %s WHERE name = %s", (quantity, item_name))
conn.commit()
cursor.close()
update_list()
# 更新库存列表
def update_list():
item_list.delete(0, tk.END)
cursor = conn.cursor()
cursor.execute("SELECT name, quantity FROM items")
inventory = cursor.fetchall()
cursor.close()
for item in inventory:
item_list.insert(tk.END, f"{item[0]}: {item[1]}")
```
5. 在GUI界面中更新库存列表
使用get_inventory函数获取库存列表,然后在GUI界面中更新列表框。
```python
# 初始化列表
update_list()
```
6. 运行程序
使用root.mainloop()运行程序,并测试功能。
```python
root.mainloop()
```
完整代码如下:
相关推荐
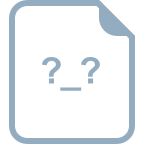
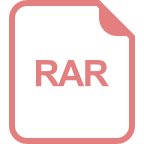
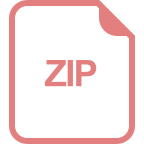















