python开发记账本课程设计
时间: 2023-10-04 12:12:42 浏览: 71
好的,下面是一个简单的Python记账本的课程设计:
## 1.需求分析
本记账本需要实现以下功能:
1. 记录每天的收入和支出。
2. 可以查询某一天或某一段时间的收支情况。
3. 可以计算某一段时间的总收入和总支出。
4. 可以输出每个月的总结报表。
## 2.设计思路
本记账本采用文件存储的方式,每一天的收支情况以及月度总结都保存在一个文本文件中。具体设计思路如下:
1. 创建一个`Record`类,用于记录每一笔收支情况,包括日期、类型(收入或支出)、金额和备注。
2. 创建一个`Account`类,用于管理所有的收支记录。这个类包括以下方法:
- `add_record(record)`:添加一条新的收支记录。
- `query(date)`:查询某一天的收支情况。
- `query_range(start_date, end_date)`:查询某一段时间的收支情况。
- `total_income(start_date, end_date)`:计算某一段时间的总收入。
- `total_expense(start_date, end_date)`:计算某一段时间的总支出。
- `report_monthly(year, month)`:生成某个月的总结报表。
3. 创建一个`FileManager`类,用于管理所有的文本文件。这个类包括以下方法:
- `get_file_name(date)`:根据日期生成对应的文件名。
- `write_record(record)`:将一条收支记录写入对应的文件中。
- `read_records(date)`:从对应的文件中读取所有的收支记录。
## 3.代码实现
下面是一个简单的Python记账本的代码实现。具体实现过程中,还需要处理一些异常情况和输入参数的格式,这里只展示主要的代码结构。
```python
import os
import datetime
class Record:
def __init__(self, date, type, amount, note):
self.date = date
self.type = type
self.amount = amount
self.note = note
class Account:
def __init__(self):
self.records = []
def add_record(self, record):
self.records.append(record)
def query(self, date):
result = []
for record in self.records:
if record.date == date:
result.append(record)
return result
def query_range(self, start_date, end_date):
result = []
for record in self.records:
if start_date <= record.date <= end_date:
result.append(record)
return result
def total_income(self, start_date, end_date):
total = 0
for record in self.records:
if record.type == 'income' and start_date <= record.date <= end_date:
total += record.amount
return total
def total_expense(self, start_date, end_date):
total = 0
for record in self.records:
if record.type == 'expense' and start_date <= record.date <= end_date:
total += record.amount
return total
def report_monthly(self, year, month):
start_date = datetime.date(year, month, 1)
end_date = datetime.date(year, month, 1) + datetime.timedelta(days=30)
income = self.total_income(start_date, end_date)
expense = self.total_expense(start_date, end_date)
balance = income - expense
print(f'{year}年{month}月总收入:{income}元')
print(f'{year}年{month}月总支出:{expense}元')
print(f'{year}年{month}月结余:{balance}元')
class FileManager:
def __init__(self, data_dir):
self.data_dir = data_dir
def get_file_name(self, date):
return os.path.join(self.data_dir, f'{date}.txt')
def write_record(self, record):
file_name = self.get_file_name(record.date)
with open(file_name, 'a') as f:
f.write(f'{record.type},{record.amount},{record.note}\n')
def read_records(self, date):
file_name = self.get_file_name(date)
if not os.path.exists(file_name):
return []
records = []
with open(file_name, 'r') as f:
for line in f:
parts = line.strip().split(',')
type = parts[0]
amount = float(parts[1])
note = parts[2]
record = Record(date, type, amount, note)
records.append(record)
return records
```
## 4.使用示例
下面是一个简单的使用示例,展示了如何创建一个记账本、添加记录、查询记录、计算总收支等功能。
```python
data_dir = '/path/to/data'
file_manager = FileManager(data_dir)
account = Account()
# 添加记录
record1 = Record(date=datetime.date(2021, 1, 1), type='income', amount=1000, note='工资')
record2 = Record(date=datetime.date(2021, 1, 3), type='expense', amount=200, note='餐饮')
record3 = Record(date=datetime.date(2021, 1, 5), type='expense', amount=300, note='购物')
file_manager.write_record(record1)
file_manager.write_record(record2)
file_manager.write_record(record3)
account.add_record(record1)
account.add_record(record2)
account.add_record(record3)
# 查询记录
records = account.query(datetime.date(2021, 1, 3))
for record in records:
print(record.date, record.type, record.amount, record.note)
# 计算总收支
total_income = account.total_income(datetime.date(2021, 1, 1), datetime.date(2021, 1, 31))
total_expense = account.total_expense(datetime.date(2021, 1, 1), datetime.date(2021, 1, 31))
print('总收入:', total_income)
print('总支出:', total_expense)
# 生成月度总结报表
account.report_monthly(2021, 1)
```
相关推荐
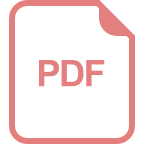















