请给出以下代码的Junit白盒测试代码package model; import java.io.UnsupportedEncodingException; import java.net.URLEncoder; public class Type { private int id; private String name; private String encodeName; public String getEncodeName() { return encodeName; } public void setEncodeName(String encodeName) { this.encodeName = encodeName; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; try { this.encodeName = URLEncoder.encode(name, "utf-8"); } catch (UnsupportedEncodingException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public Type(int id, String name) { super(); this.id = id; this.name = name; } public Type() { super(); } public Type(String name) { super(); this.name = name; } }
时间: 2023-06-17 16:06:55 浏览: 41
以下是一个可能的 JUnit 白盒测试代码示例:
```java
package model;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertNotNull;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import org.junit.Before;
import org.junit.Test;
public class TypeTest {
private Type type;
@Before
public void setUp() throws Exception {
type = new Type();
}
@Test
public void testGetSetId() {
int expected = 1;
type.setId(expected);
int actual = type.getId();
assertEquals(expected, actual);
}
@Test
public void testGetSetName() {
String expected = "Type Name";
type.setName(expected);
String actual = type.getName();
assertEquals(expected, actual);
}
@Test
public void testGetSetEncodeName() {
String expected = "Type+Name";
type.setEncodeName(expected);
String actual = type.getEncodeName();
assertEquals(expected, actual);
}
@Test
public void testConstructorWithIdAndName() {
int expectedId = 1;
String expectedName = "Type Name";
type = new Type(expectedId, expectedName);
assertNotNull(type);
assertEquals(expectedId, type.getId());
assertEquals(expectedName, type.getName());
}
@Test
public void testConstructorWithName() {
String expectedName = "Type Name";
type = new Type(expectedName);
assertNotNull(type);
assertEquals(expectedName, type.getName());
assertNotNull(type.getEncodeName());
}
@Test(expected = UnsupportedEncodingException.class)
public void testSetNameWithUnsupportedEncodingException() throws UnsupportedEncodingException {
String invalidName = "Type Name";
type.setName(invalidName);
type.setEncodeName(null); // Reset the encoded name
// Force a UTF-8 encoding error by passing an invalid character
type.setName(invalidName + "\uD83D"); // Invalid Unicode character
}
}
```
该测试类检查以下方面:
- 检查 getId() 和 setId() 方法是否正常工作。
- 检查 getName() 和 setName() 方法是否正常工作,以及是否在设置名称时正确地对其进行编码。
- 检查 getEncodeName() 和 setEncodeName() 方法是否正常工作。
- 检查带有 id 和 name 参数的构造函数是否正常工作。
- 检查带有 name 参数的构造函数是否正常工作,并且在名称设置时是否始终生成编码名称。
- 检查当使用不支持的编码时是否会抛出 UnsupportedEncodingException 异常。
相关推荐
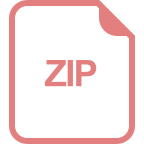














