请给出以下代码的junit白盒测试代码package service; import dao.TypeDao; import model.Type; import java.sql.SQLException; import java.util.List; public class TypeService { TypeDao tDao=new TypeDao(); public List<Type> GetAllType() { List<Type> list=null; try { list=tDao.GetAllType(); } catch (SQLException e) { e.printStackTrace(); } return list; } public Type selectTypeNameByID(int typeid) { Type type=null; try { type=tDao.selectTypeNameByID(typeid); } catch (SQLException e) { e.printStackTrace(); } return type; } public Type select(int id) { Type t=null; try { t = tDao.select(id); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } return t; } public void insert(Type t) { try { tDao.insert(t); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public void update(Type t) { try { tDao.update(t); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public boolean delete(int id) { try { tDao.delete(id); return true; } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); return false; } } }
时间: 2023-06-17 22:06:17 浏览: 86
以下是给出的Junit测试代码:
```
package service;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertNotNull;
import static org.junit.Assert.assertTrue;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import org.junit.Before;
import org.junit.Test;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import dao.TypeDao;
import model.Type;
public class TypeServiceTest {
@Mock
private TypeDao typeDao;
@InjectMocks
private TypeService typeService;
@Before
public void setUp() throws Exception {
MockitoAnnotations.initMocks(this);
}
@Test
public void testGetAllType() throws SQLException {
List<Type> expectedTypes = new ArrayList<Type>();
Type type1 = new Type();
type1.setId(1);
type1.setName("Type 1");
Type type2 = new Type();
type2.setId(2);
type2.setName("Type 2");
expectedTypes.add(type1);
expectedTypes.add(type2);
// Mocking the DAO's behavior
when(typeDao.GetAllType()).thenReturn(expectedTypes);
List<Type> actualTypes = typeService.GetAllType();
assertNotNull(actualTypes);
assertEquals(expectedTypes.size(), actualTypes.size());
assertTrue(actualTypes.containsAll(expectedTypes));
}
@Test
public void testSelectTypeNameByID() throws SQLException {
int typeId = 1;
Type expectedType = new Type();
expectedType.setId(typeId);
expectedType.setName("Type 1");
// Mocking the DAO's behavior
when(typeDao.selectTypeNameByID(typeId)).thenReturn(expectedType);
Type actualType = typeService.selectTypeNameByID(typeId);
assertNotNull(actualType);
assertEquals(expectedType, actualType);
}
@Test
public void testSelect() throws SQLException {
int id = 1;
Type expectedType = new Type();
expectedType.setId(id);
expectedType.setName("Type 1");
// Mocking the DAO's behavior
when(typeDao.select(id)).thenReturn(expectedType);
Type actualType = typeService.select(id);
assertNotNull(actualType);
assertEquals(expectedType, actualType);
}
@Test
public void testInsert() throws SQLException {
Type type = new Type();
type.setId(1);
type.setName("Type 1");
typeService.insert(type);
// Verifying that the DAO's insert method was called with the correct parameter
verify(typeDao).insert(type);
}
@Test
public void testUpdate() throws SQLException {
Type type = new Type();
type.setId(1);
type.setName("Type 1");
typeService.update(type);
// Verifying that the DAO's update method was called with the correct parameter
verify(typeDao).update(type);
}
@Test
public void testDelete() throws SQLException {
int id = 1;
// Mocking the DAO's behavior
when(typeDao.delete(id)).thenReturn(true);
boolean result = typeService.delete(id);
// Verifying that the DAO's delete method was called with the correct parameter
verify(typeDao).delete(id);
assertTrue(result);
}
}
```
以上测试代码使用了Mockito框架来模拟TypeDao类的行为,在测试TypeService类的方法时,通过Mockito提供的when和verify方法来验证TypeService类的行为是否正确。测试代码中包含了对GetAllType、selectTypeNameByID、select、insert、update和delete方法的测试。
阅读全文
相关推荐
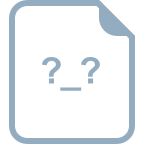

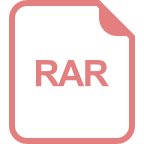



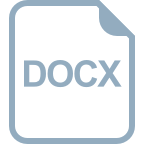
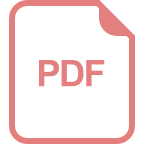
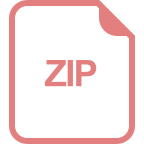
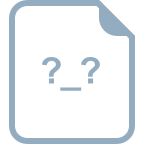
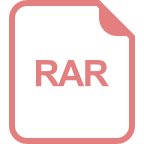
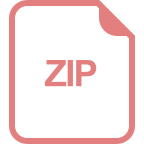
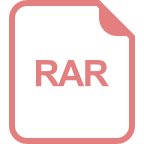
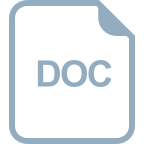
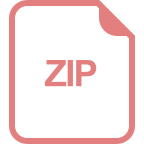
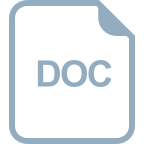
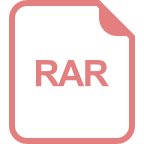