java根据经纬度获取所在区域
时间: 2023-08-12 21:06:37 浏览: 135
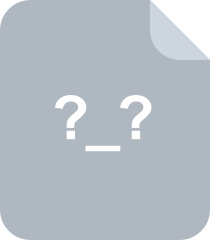
根据经纬度获取地理位置

要根据经纬度获取所在区域,可以使用反向地理编码(Reverse Geocoding)技术。Java中可以使用一些开源库来实现这个功能,比如 GeoTools、JTS Topology Suite、Gisgraphy 等等。
其中,Gisgraphy 是一个基于开放数据的反向地理编码服务,支持多种数据格式和多种编程语言,包括 Java。你可以通过调用 Gisgraphy 的 API 来获取经纬度所在的区域信息,比如国家、省份、城市、街道等等。
以下是一个使用 Gisgraphy 的 Java 示例代码:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
public class ReverseGeocoding {
public static void main(String[] args) {
try {
// 经纬度
double lat = 31.2304;
double lon = 121.4737;
// 调用 Gisgraphy 的 API
String url = "http://services.gisgraphy.com/reversegeocoding/search?lat=" +
lat + "&lon=" + lon + "&format=json&placetype=all&from=1&to=1";
HttpURLConnection conn = (HttpURLConnection) new URL(url).openConnection();
conn.setRequestMethod("GET");
// 获取响应结果
BufferedReader reader = new BufferedReader(new InputStreamReader(conn.getInputStream()));
StringBuilder sb = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
sb.append(line);
}
reader.close();
// 解析 JSON 格式的响应结果
String json = sb.toString();
String country = json.substring(json.indexOf("\"name\":\"") + 8, json.indexOf("\",\"type\":\"COUNTRY\""));
String state = json.substring(json.indexOf("\"name\":\"", json.indexOf("\"type\":\"STATE\"")) + 8, json.indexOf("\",\"type\":\"STATE\""));
String city = json.substring(json.indexOf("\"name\":\"", json.indexOf("\"type\":\"CITY\"")) + 8, json.indexOf("\",\"type\":\"CITY\""));
String street = json.substring(json.indexOf("\"name\":\"", json.indexOf("\"type\":\"STREET\"")) + 8, json.indexOf("\",\"type\":\"STREET\""));
System.out.println("Country: " + country);
System.out.println("State: " + state);
System.out.println("City: " + city);
System.out.println("Street: " + street);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在上面的代码中,我们首先构造了一个 Gisgraphy 的 API 请求 URL,将经纬度和其他参数拼接在 URL 中。然后发送 HTTP GET 请求,获取响应结果并解析出国家、省份、城市、街道等信息。最后输出这些信息到控制台。注意,在实际使用中,你需要替换掉自己的 Gisgraphy API KEY 和经纬度值。
阅读全文
相关推荐

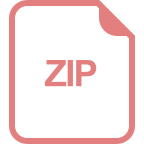
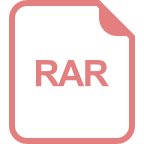
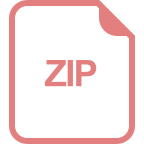


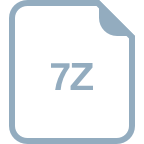

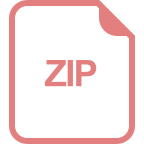
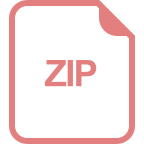
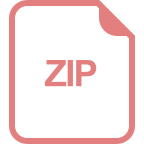
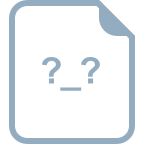
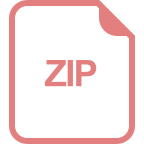