往链表中输入任意个数字并打印出来,用c++写
时间: 2024-10-07 07:05:45 浏览: 31
在C++中,我们可以使用链表数据结构来存储任意个数字,并通过迭代遍历链表的方式将它们打印出来。这里是一个简单的示例,我们首先创建一个节点类`Node`,然后定义一个链表类`LinkedList`,并在其中包含插入元素和打印链表的方法。
```cpp
#include <iostream>
// 定义链表节点
class Node {
public:
int data;
Node* next;
// 构造函数
Node(int value) : data(value), next(nullptr) {}
};
// 链表类
class LinkedList {
private:
Node* head;
public:
// 初始化链表为空
LinkedList() : head(nullptr) {}
// 插入节点
void insert(int value) {
if (head == nullptr) {
head = new Node(value);
} else {
Node* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = new Node(value);
}
}
// 打印链表
void printList() {
Node* temp = head;
while (temp != nullptr) {
std::cout << temp->data << " ";
temp = temp->next;
}
std::cout << std::endl;
}
};
int main() {
LinkedList list;
int input;
std::cout << "请输入数字(输入0结束):" << std::endl;
while (std::cin >> input && input != 0) {
list.insert(input);
}
list.printList(); // 打印链表中的所有数字
return 0;
}
```
在这个程序中,用户可以逐个输入数字直到输入0为止。每输入一个数字,就会自动添加到链表中,最后通过`printList()`函数输出链表中的所有数字。
阅读全文
相关推荐

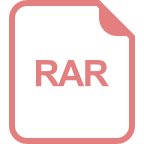



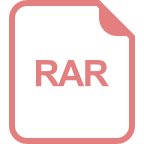













