#include <stdio.h> #define MaxSize[100+5] typedef int ElemType; typedef struct { ElemType a[MaxSize]; int n; }SeqList;纠正
时间: 2024-10-02 21:03:33 浏览: 51
在C语言中,`#include <stdio.h>`是一个预处理指令,用于引入标准输入输出库,以便使用printf和scanf等函数。接下来的部分是一些自定义声明:
- `MaxSize` 使用了宏定义,表示一个最大数组大小,这里将其设置为100加5。注意,宏定义通常不需要方括号[],因为它是替换而非变量分配。
- `typedef` 是一种类型别名关键字,它允许我们给已存在的数据类型起一个新的名字。在这里:
- `ElemType` 定义了一个新的类型名,可能是整型(int)或其他支持的数据类型。
- `struct SeqList` 是一个结构体类型的定义,包含了两个成员:
- `a` 是一个`ElemType`类型的数组,长度由`MaxSize`定义。
- `n` 是一个整型变量,可能代表序列列表中元素的数量。
正确的语法应该是去掉数组`a`的方括号,因为宏定义不应用于数组大小。修正后的代码应如下所示:
```c
#include <stdio.h>
// 宏定义数组的最大尺寸
#define MaxSize 100 + 5
// 类型别名
typedef int ElemType;
// 结构体 SeqList 的定义
typedef struct {
ElemType a[MaxSize]; // 移除多余的 []
int n;
} SeqList;
```
相关问题
#include <stdio.h> #include <stdlib.h> #define MAXSIZE 100 typedef int ElemType; typedef struct
这段代码是C语言中的头文件引用和一些定义的示例。其中,`#include <stdio.h>`和`#include <stdlib.h>`是用来引入标准库函数的头文件。`stdio.h`包含了输入输出相关的函数,如`printf`和`scanf`;`stdlib.h`包含了一些常用的函数,如内存分配函数`malloc`和随机数生成函数`rand`。
`#define MAXSIZE 100`是一个宏定义,用来定义一个常量`MAXSIZE`,它的值为100。
`typedef int ElemType;`是用来给`int`类型起一个别名`ElemType`,方便在代码中使用。
至于代码中的结构体部分,由于你没有提供完整的代码,所以无法给出具体的解释。但一般来说,结构体用于定义一种自定义的数据类型,可以包含多个不同类型的成员变量。
补全#include<stdio.h> #include<malloc.h> #define MaxSize 50 typedef int ElemType; typedef struct { Elem
#include<stdio.h>
#include<malloc.h>
#define MaxSize 50
typedef int ElemType;
typedef struct
{
ElemType data[MaxSize];
int length;
}SqList;
这段代码是一个C语言的头文件补全,包含了stdio.h和malloc.h两个头文件,并定义了一个常量MaxSize为50。同时,定义了一个类型别名ElemType为int,并声明了一个结构体SqList,其中包含了一个数组data和一个整型变量length。
阅读全文
相关推荐
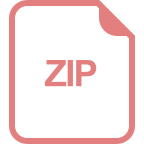
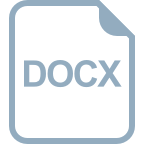
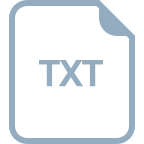














