import axios from 'axios'; // 创建一个取消请求的 CancelToken const cancelToken = axios.CancelToken; let cancel; // 创建一个 Axios 实例 const request = axios.create(); // 请求拦截器 request.interceptors.request.use(config => { // 在每个请求中设置取消请求的 token config.cancelToken = new cancelToken(function executor(c) { cancel = c; }); // 其他请求拦截逻辑... return config; }, err => { return Promise.reject(err); }); // 取消所有请求的方法 function cancelAllRequests() { cancel('取消所有请求'); } // 使用示例 request.get('/api/data1') .then(response => { // 请求成功的处理逻辑 }) .catch(error => { // 请求失败的处理逻辑 }); request.get('/api/data2') .then(response => { // 请求成功的处理逻辑 }) .catch(error => { // 请求失败的处理逻辑 }); // 取消所有请求 cancelAllRequests(); 报Uncaught TypeError: cancel is not a function
时间: 2024-04-10 13:33:01 浏览: 21
根据你提供的代码,报错"Uncaught TypeError: cancel is not a function"是因为在调用`cancelAllRequests()`方法时,`cancel`变量没有被正确赋值为取消请求的函数。
在请求拦截器中,你使用了`cancel`变量来保存取消请求的函数,但是在定义`cancelAllRequests()`方法时,没有将`cancel`作为参数传入。因此,`cancel`变量在`cancelAllRequests()`方法中是未定义的。
要解决这个问题,你可以将`cancel`作为参数传递给`cancelAllRequests()`方法,确保在调用该方法时,`cancel`变量被正确传递进去。修改后的代码如下:
```javascript
// 取消所有请求的方法
function cancelAllRequests(cancel) {
cancel('取消所有请求');
}
// 使用示例
request.get('/api/data1')
.then(response => {
// 请求成功的处理逻辑
})
.catch(error => {
// 请求失败的处理逻辑
});
request.get('/api/data2')
.then(response => {
// 请求成功的处理逻辑
})
.catch(error => {
// 请求失败的处理逻辑
});
// 取消所有请求
cancelAllRequests(cancel);
```
通过将`cancel`作为参数传递给`cancelAllRequests()`方法,可以解决"Uncaught TypeError: cancel is not a function"错误。
相关推荐
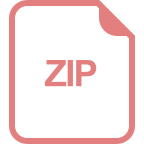
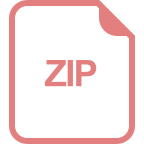
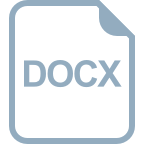















