请你写一段c语言代码实现解密过程
时间: 2024-09-28 21:14:45 浏览: 37
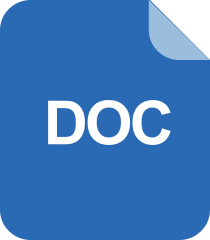
C语言 加密解密 课程设计
在 C 语言中,解密 ROT_Encrypt 加密的方法可以这样编写,假设我们已经知道加密的关键值(这里为了简化例子,关键值设为14):
```c
#include <stdio.h>
#include <string.h>
// 假设明文ASCII码范围
#define MAX_CHAR 94
void decrypt_rot14(char* encrypted_text, char* plaintext) {
int len = strlen(encrypted_text);
for (int i = 0; i < len; ++i) {
// 解密:从14+33(加后取模94)还原到14,并加上32将小写转为大写
unsigned char decrypted_char = (encrypted_text[i] - 33 - 14) % MAX_CHAR;
// 需要注意的是,此处仅适用于小写字母的解密,对于非字母字符不做处理
if ('a' <= decrypted_char && decrypted_char <= 'z') {
decrypted_char += 32; // 转换为大写字母
}
plaintext[i] = decrypted_char;
}
}
int main() {
char encrypted[] = "Your Encrypted Text Here";
char decrypted[50]; // 假设最多需要50个字符的解密文本
decrypt_rot14((char*)encrypted, decrypted);
printf("Decrypted text: %s\n", decrypted);
return 0;
}
```
这只是一个基础的解密示例,实际应用中可能还需要考虑字符的边界检查、非字母字符的处理以及更复杂的加密算法。运行这个程序会输出解密后的文本。记得替换 `Your Encrypted Text Here` 为你需要解密的实际内容。
阅读全文
相关推荐
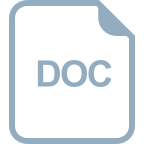
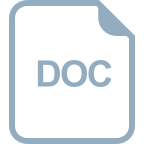
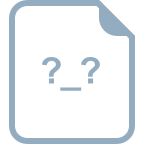
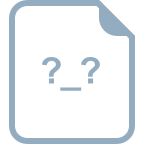
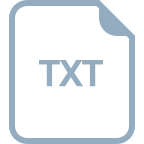
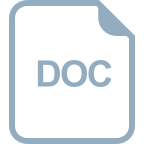
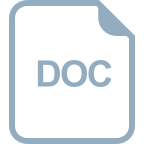
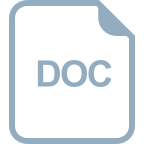
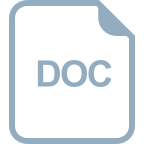
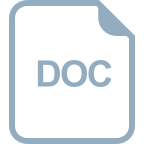







