写一个程序,在输入的句子中只选择字母,按照字母顺序排列后输出,只输入在长度八十以下的一个句子,对于统一的罗马数字小写字母要排在大写字母前面这道题怎么用c语言去表示用c语言怎么去进行韩语编写
时间: 2024-09-26 19:13:37 浏览: 19
要用C语言解决这个问题,你可以遵循以下步骤:
首先,定义一个函数来清理和整理输入的句子,保留字母并区分大小写:
```c
#include <ctype.h>
#include <stdio.h>
char *clean_and_sort(const char *input) {
int strLength = strlen(input);
char cleanedStr[strLength];
int j = 0;
// 遍历输入,仅保存字母,转换小写字母到前面
for (int i = 0; i < strLength; i++) {
if (isalpha(input[i])) {
if (islower(input[i])) {
cleanedStr[j++] = tolower(input[i]);
} else {
while (j > 0 && cleanedStr[j - 1] <= input[i]) { // 如果存在比当前更大的小写字母,交换
cleanedStr[j] = cleanedStr[j - 1];
j--;
}
cleanedStr[j++] = input[i];
}
}
}
cleanedStr[j] = '\0'; // 结束字符串并添加空字符
return cleanedStr;
}
int main() {
char input[81]; // 限制输入字符串最长为80字符
printf("Enter a sentence (max 80 characters): ");
fgets(input, sizeof(input), stdin); // 获取用户的输入
input[strcspn(input, "\n")] = '\0'; // 清除换行符
printf("Sorted and cleaned alphabet from input: %s\n", clean_and_sort(input));
return 0;
}
```
如果要使用C语言实现韩语处理功能(通常韩语有其特殊的ASCII映射),C库的标准处理可能不足以应对这种需求,因为韩语字母表和普通ASCII有所不同。在C++11及以上版本可以尝试使用ICU (International Components for Unicode),但这是一个复杂的话题。
相关推荐
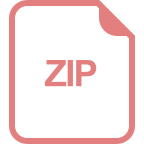
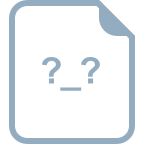













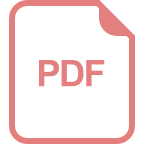
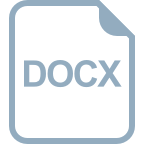
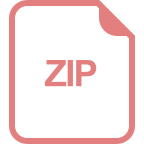