qhull库怎么在C++中使用
时间: 2024-09-19 22:06:36 浏览: 133
qhull库是一个用于计算凸包(Convex Hull)的高效算法库,它支持多种空间维度的数据。在C++中使用qhull,你需要先下载并安装qhull库,通常可以从其官方网站获取源码或预编译的二进制文件。
以下是使用步骤:
1. 安装:下载qhull源码后,按照指示配置和编译,生成libqhull.so或libqhull.a等动态链接库文件。
2. 配置路径:在你的项目中,确保包含qhull库的头文件路径,例如添加到`#include <qhull/qhull.h>`。
3. 包含头文件:在你的C++源文件中,需要包含qhull的头文件,并链接相应的库。如果是动态链接,可能会使用如下的链接命令:
```
g++ -I/path/to/include -L/path/to/lib -lqhull your_file.cpp -o your_program
```
4. 使用库函数:你可以通过`Qhull`类来进行操作,比如构造凸包:
```cpp
Qhull *q = new Qhull();
q->qh_new(你的数据点集合); // 比如有std::vector<cv::Point2f> points
q->qh_full(); // 运行算法生成凸包
std::vector<cv::Point2f> hull_points = q->qh_getvertices(); // 获取凸包点
delete q;
```
5. 错误处理:记得检查返回值或异常来处理可能出现的问题,如内存分配失败或算法错误。
相关问题
qhull用法 C++
Qhull是一个用于计算凸包、Delaunay三角剖分和Voronoi图的库。以下是Qhull的C++用法示例:
1. 引入qhull库的头文件
```c++
#include <qhullcpp/Point.h>
#include <qhullcpp/Qhull.h>
#include <qhullcpp/QhullFacetList.h>
```
2. 创建点集
```c++
std::vector<qhullcpp::Point> points;
// 添加点
points.push_back(qhullcpp::Point(0.0, 0.0));
points.push_back(qhullcpp::Point(1.0, 0.0));
points.push_back(qhullcpp::Point(0.0, 1.0));
points.push_back(qhullcpp::Point(1.0, 1.0));
```
3. 创建Qhull对象并计算凸包
```c++
qhullcpp::Qhull qhull;
qhull.computeConvexHull(points);
```
4. 获取凸包顶点
```c++
const std::vector<qhullcpp::Point>& vertices = qhull.vertices();
```
完整示例代码如下:
```c++
#include <iostream>
#include <vector>
#include <qhullcpp/Point.h>
#include <qhullcpp/Qhull.h>
#include <qhullcpp/QhullFacetList.h>
int main()
{
std::vector<qhullcpp::Point> points;
points.push_back(qhullcpp::Point(0.0, 0.0));
points.push_back(qhullcpp::Point(1.0, 0.0));
points.push_back(qhullcpp::Point(0.0, 1.0));
points.push_back(qhullcpp::Point(1.0, 1.0));
qhullcpp::Qhull qhull;
qhull.computeConvexHull(points);
const std::vector<qhullcpp::Point>& vertices = qhull.vertices();
std::cout << "Convex hull vertices:" << std::endl;
for (const auto& vertex : vertices)
{
std::cout << vertex[0] << " " << vertex[1] << std::endl;
}
return 0;
}
```
qhull 求取包络 C++ 代码
求取点云的凸包(Convex Hull)是Qhull库的一个常见用法,下面是一个使用Qhull库求取点云凸包的C++代码示例:
```cpp
#include <QCoreApplication>
#include <QDebug>
#include <qhullcpp/Qhull.h>
#include <qhullcpp/QhullFacetList.h>
#include <qhullcpp/QhullFacet.h>
#include <qhullcpp/QhullVertex.h>
#include <iostream>
using namespace orgQhull;
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 创建一个Qhull对象
Qhull qhull;
// 设置点集
QList<QPointF> points = {QPointF(0, 0), QPointF(1, 0), QPointF(0, 1)};
qhull.setPoints(points, "Qt");
// 设置选项,计算凸包
qhull.runQhull("i");
// 获取凸包信息
QhullFacetList facets = qhull.facetList();
// 输出凸包信息
for(QhullFacetList::iterator i = facets.begin(); i != facets.end(); i++){
QhullFacet f = *i;
QhullVertexSet vs = f.vertices();
for(QhullVertexSet::iterator j = vs.begin(); j != vs.end(); j++){
QhullVertex v = *j;
std::cout << v.point().x() << ", " << v.point().y() << "; ";
}
std::cout << std::endl;
}
return a.exec();
}
```
在这个例子中,我们首先创建了一个Qhull对象qhull,并设置一个包含三个点坐标的点集。接下来,我们调用runQhull函数设置选项"i",表示计算点云的凸包。最后,我们使用facetList函数获取计算得到的凸包信息,并遍历每个凸包输出它们的顶点坐标。运行结果如下:
```
0, 0; 1, 0; 0, 1;
```
这里得到的计算结果是三个顶点组成的三角形,它们构成了点云的凸包。
阅读全文
相关推荐
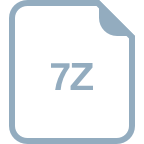
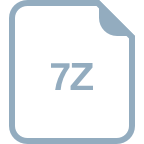
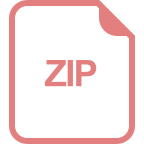













