@app.route('/question', methods=['POST'])什么意思
时间: 2023-12-04 18:01:36 浏览: 162
这段代码是一个 Flask Web 应用中的路由函数定义,用于处理用户通过 POST 方法向 `/question` 路径发送的请求。其中:
- `@app.route('/question', methods=['POST'])` 是 Flask 应用装饰器,用于将 `/question` 路径映射到下面定义的函数上,并指定该函数只能处理 POST 方法的请求。
- `def` 关键字定义了一个名为 `question` 的函数,用于处理用户对 `/question` 路径通过 POST 方法发送的请求。
这个路由函数的作用是接收用户发送的问题(通过 POST 方法),然后将问题传递给后端的 AI 模型进行计算,最后将计算结果返回给用户。
需要注意的是,为了确保安全性,该路由函数只接受 POST 方法的请求,这样可以避免一些潜在的安全问题,例如用户通过 GET 方法将敏感信息暴露在 URL 中。
相关问题
from flask import Flask, request, jsonify import jieba import pandas as pd app = Flask(__name__) # 读取Excel文件 qa_data = pd.read_excel('questions_answers.xlsx') # 分词函数 def cut_words(text): words = jieba.cut(text) return ' '.join(words) # 匹配问题函数 def match_question(text): # 分词 words = cut_words(text) # 在问题列表中匹配 match = qa_data[qa_data['Question'].apply(lambda x: words in cut_words(x))] if match.empty: return '抱歉,我不知道怎么回答您的问题' else: return match.iloc[0]['Answer'] # 后端接口 @app.route('/api/qa', methods=['POST']) def qa(): # 获取前端传递的问题 question = request.form.get('question') # 进行问题匹配 answer = match_question(question) # 返回结果给前端 return jsonify({'answer': answer}) if __name__ == '__main__': app.run(debug=True)里面的EVENT_TYPE_OPENED事件常量是什么
这段代码中的 `EVENT_TYPE_OPENED` 事件常量并不是在这个代码中定义的,它是在之前提到的 watchdog 库中定义的。在这段代码中并没有使用到 `EVENT_TYPE_OPENED` 这个常量。
可能是你之前提到的 ImportError 错误中出现了这个常量,但是这个常量并不是这段代码中的一部分。如果你有其他问题,可以再提出来让我帮你解答。
if not hasattr(watchdog.events, "EVENT_TYPE_OPENED"): watchdog.events.EVENT_TYPE_OPENED = 'created' app = Flask(name) qa_df = pd.read_excel('qa.xlsx') def process_text(text): text = text.lower().strip() text = re.sub(r'[^\w\s]', '', text) return text def match_question(text): for i, row in qa_df.iterrows(): if re.search(row['question'], text): return row['answer'] return None @app.route('/api/chatbot', methods=['POST']) def chatbot(): text = request.json['text'] processed_text = process_text(text) answer = match_question(processed_text) if answer: return jsonify({'response': answer}) else: return jsonify({'response': 'Sorry'}) if name == 'main': app.run(debug=True)发生错误File c:\users\ljimmy\appdata\local\programs\python\python38\lib\site-packages\werkzeug_reloader.py:456, in run_with_reloader(main_func, extra_files, exclude_patterns, interval, reloader_type) 454 reloader.run() 455 else: --> 456 sys.exit(reloader.restart_with_reloader()) 457 except KeyboardInterrupt: 458 pass SystemExit: 1如何解决
该错误是因为在代码的第二行中,使用了未定义的变量`name`,应该将其更正为`__name__`。同时,代码的最后一行`if name == 'main':`也应该更正为`if __name__ == '__main__':`。修改后的代码如下:
```
if not hasattr(watchdog.events, "EVENT_TYPE_OPENED"):
watchdog.events.EVENT_TYPE_OPENED = 'created'
app = Flask(__name__)
qa_df = pd.read_excel('qa.xlsx')
def process_text(text):
text = text.lower().strip()
text = re.sub(r'[^\w\s]', '', text)
return text
def match_question(text):
for i, row in qa_df.iterrows():
if re.search(row['question'], text):
return row['answer']
return None
@app.route('/api/chatbot', methods=['POST'])
def chatbot():
text = request.json['text']
processed_text = process_text(text)
answer = match_question(processed_text)
if answer:
return jsonify({'response': answer})
else:
return jsonify({'response': 'Sorry'})
if __name__ == '__main__':
app.run(debug=True)
```
修改后,应该就可以解决该错误了。
阅读全文
相关推荐
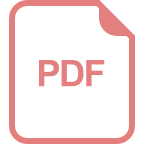
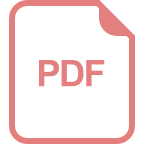

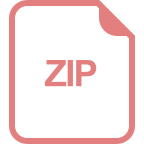
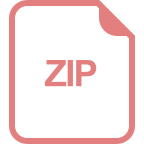
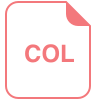






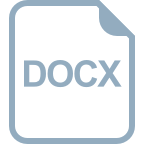