STM32 按键控制数码管
时间: 2023-10-18 10:04:40 浏览: 421
要用 STM32 控制数码管,需要先连接好 STM32 和数码管,并且要对 STM32 的 GPIO 端口进行配置。以下是一个简单的按键控制数码管的代码示例:
```c
#include "stm32f10x.h"
#define LED_GPIO GPIOC
#define LED_PIN GPIO_Pin_13
#define BUTTON_GPIO GPIOA
#define BUTTON_PIN GPIO_Pin_0
#define SEGMENT_GPIO GPIOB
#define SEGMENT_PIN_0 GPIO_Pin_0
#define SEGMENT_PIN_1 GPIO_Pin_1
#define SEGMENT_PIN_2 GPIO_Pin_5
#define SEGMENT_PIN_3 GPIO_Pin_6
#define SEGMENT_PIN_4 GPIO_Pin_7
#define SEGMENT_PIN_5 GPIO_Pin_8
#define SEGMENT_PIN_6 GPIO_Pin_9
#define SEGMENT_PIN_7 GPIO_Pin_10
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
/* LED GPIO pin configuration */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
GPIO_InitStructure.GPIO_Pin = LED_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_GPIO, &GPIO_InitStructure);
/* Button GPIO pin configuration */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = BUTTON_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(BUTTON_GPIO, &GPIO_InitStructure);
/* Segment GPIO pin configuration */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = SEGMENT_PIN_0 | SEGMENT_PIN_1 | SEGMENT_PIN_2 | SEGMENT_PIN_3 |
SEGMENT_PIN_4 | SEGMENT_PIN_5 | SEGMENT_PIN_6 | SEGMENT_PIN_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(SEGMENT_GPIO, &GPIO_InitStructure);
}
int main(void)
{
GPIO_Configuration();
uint8_t segment_num = 0;
while (1)
{
if (GPIO_ReadInputDataBit(BUTTON_GPIO, BUTTON_PIN) == RESET)
{
/* Button is pressed */
LED_GPIO->BRR = LED_PIN; /* Turn off LED */
segment_num++; /* Increment segment number */
if (segment_num > 9)
{
segment_num = 0;
}
}
else
{
/* Button is not pressed */
LED_GPIO->BSRR = LED_PIN; /* Turn on LED */
}
/* Display segment number on the 7-segment display */
switch (segment_num)
{
case 0:
SEGMENT_GPIO->ODR = SEGMENT_PIN_0 | SEGMENT_PIN_1 | SEGMENT_PIN_2 | SEGMENT_PIN_3 |
SEGMENT_PIN_4 | SEGMENT_PIN_5;
break;
case 1:
SEGMENT_GPIO->ODR = SEGMENT_PIN_1 | SEGMENT_PIN_2;
break;
case 2:
SEGMENT_GPIO->ODR = SEGMENT_PIN_0 | SEGMENT_PIN_1 | SEGMENT_PIN_3 | SEGMENT_PIN_4 |
SEGMENT_PIN_6;
break;
case 3:
SEGMENT_GPIO->ODR = SEGMENT_PIN_0 | SEGMENT_PIN_1 | SEGMENT_PIN_2 | SEGMENT_PIN_3 |
SEGMENT_PIN_6;
break;
case 4:
SEGMENT_GPIO->ODR = SEGMENT_PIN_1 | SEGMENT_PIN_2 | SEGMENT_PIN_5 | SEGMENT_PIN_6;
break;
case 5:
SEGMENT_GPIO->ODR = SEGMENT_PIN_0 | SEGMENT_PIN_2 | SEGMENT_PIN_3 | SEGMENT_PIN_5 |
SEGMENT_PIN_6;
break;
case 6:
SEGMENT_GPIO->ODR = SEGMENT_PIN_0 | SEGMENT_PIN_2 | SEGMENT_PIN_3 | SEGMENT_PIN_4 |
SEGMENT_PIN_5 | SEGMENT_PIN_6;
break;
case 7:
SEGMENT_GPIO->ODR = SEGMENT_PIN_0 | SEGMENT_PIN_1 | SEGMENT_PIN_2;
break;
case 8:
SEGMENT_GPIO->ODR = SEGMENT_PIN_0 | SEGMENT_PIN_1 | SEGMENT_PIN_2 | SEGMENT_PIN_3 |
SEGMENT_PIN_4 | SEGMENT_PIN_5 | SEGMENT_PIN_6;
break;
case 9:
SEGMENT_GPIO->ODR = SEGMENT_PIN_0 | SEGMENT_PIN_1 | SEGMENT_PIN_2 | SEGMENT_PIN_3 |
SEGMENT_PIN_5 | SEGMENT_PIN_6;
break;
}
}
}
```
这个代码示例使用 PA0 作为按键输入口,PC13 作为 LED 输出口,以及 PB0~PB7 作为数码管显示口。在循环中,如果按键被按下,那么就会将数码管上的数字加 1,同时会将 LED 关闭;如果按键没有被按下,那么就会将 LED 打开。数码管上的数字通过 switch 语句来实现控制。需要注意的是,在调用 GPIO_Init 函数之前,需要先使能对应 GPIO 端口的时钟。
阅读全文
相关推荐
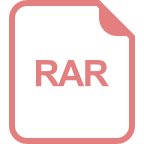
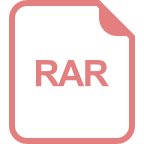







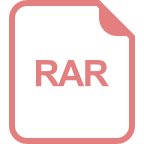
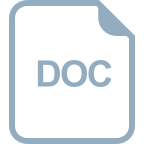




