初始化一个大堆的优先级队列
时间: 2024-09-16 13:03:18 浏览: 42
初始化一个大堆的优先级队列通常是在数据结构中使用堆这种特殊的数据结构来实现。堆是一种特殊的树形结构,其中每个节点的值都大于或等于其子节点的值(称为最大堆),或者小于或等于其子节点的值(称为最小堆)。对于优先级队列,我们通常会选择最大堆,因为我们需要快速获取队列中的最大元素。
在许多编程语言中,例如Java,我们可以使用`PriorityQueue`类来初始化一个大堆优先级队列。下面是一个简单的例子:
```java
import java.util.PriorityQueue;
// 创建一个默认的最大堆优先级队列
PriorityQueue<Integer> priorityQueue = new PriorityQueue<>();
```
如果你想要存储自定义的对象并基于某个属性进行排序,你可以创建一个实现了`Comparator`接口的比较器,并传入这个比较器:
```java
PriorityQueue<MyObject> priorityQueue = new PriorityQueue<>(new MyObjectComparator());
```
在这里,`MyObjectComparator`是一个类,它实现了`Comparator<MyObject>`,用于按照`MyObject`中的某个字段来确定优先级。
相关问题
0-1背包问题要求用分支限界法实现算法用C++ STL最小堆来实现已搜索的解状态空间结点的优先级队列
好的,我可以为您解答这个问题。
0-1背包问题是动态规划问题中的一个经典问题,其目的是在给定一组物品和一个背包容量的情况下,选择一些物品放入背包中,使得背包中物品的总价值最大。而分支限界法是一种搜索算法,它可以通过优先队列来维护搜索状态空间中的结点,从而提高搜索效率。
下面是使用C++ STL最小堆来实现已搜索的解状态空间结点的优先级队列的代码:
```c++
#include <iostream>
#include <vector>
#include <queue>
#include <algorithm>
using namespace std;
// 物品结构体
struct Item {
int weight; // 物品重量
int value; // 物品价值
};
// 结点结构体
struct Node {
int level; // 结点所在层数
int profit; // 当前背包中物品的总价值
int weight; // 当前背包中物品的总重量
vector<bool> solution; // 当前背包中物品的选择情况
double bound; // 当前结点的价值上界
bool operator<(const Node& other) const { // 重载小于号,用于STL最小堆排序
return bound < other.bound;
}
};
// 计算结点的价值上界
double calc_bound(const vector<Item>& items, const Node& node, int capacity) {
double bound = node.profit;
int weight = node.weight;
int level = node.level;
while (level < items.size() && weight + items[level].weight <= capacity) {
bound += items[level].value;
weight += items[level].weight;
level++;
}
if (level < items.size()) {
bound += (capacity - weight) * items[level].value / (double)items[level].weight;
}
return bound;
}
// 分支限界法求解0-1背包问题
int knapsack(const vector<Item>& items, int capacity) {
// 按照单位重量的价值从大到小排序
vector<int> indices(items.size());
iota(indices.begin(), indices.end(), 0);
sort(indices.begin(), indices.end(), [&items](int i, int j) {
return items[i].value * 1.0 / items[i].weight > items[j].value * 1.0 / items[j].weight;
});
// 初始化根结点
Node root = {0, 0, 0, vector<bool>(items.size(), 0), 0};
root.bound = calc_bound(items, root, capacity);
// 初始化最小堆
priority_queue<Node> Q;
Q.push(root);
// 开始搜索
int max_profit = 0;
while (!Q.empty()) {
Node cur = Q.top();
Q.pop();
if (cur.bound < max_profit) {
continue;
}
if (cur.level == items.size()) {
max_profit = max(max_profit, cur.profit);
continue;
}
// 选择当前物品
Node left = cur;
left.level++;
left.weight += items[indices[left.level - 1]].weight;
left.profit += items[indices[left.level - 1]].value;
left.solution[indices[left.level - 1]] = true;
if (left.weight <= capacity) {
left.bound = calc_bound(items, left, capacity);
if (left.bound > max_profit) {
Q.push(left);
}
}
// 不选择当前物品
Node right = cur;
right.level++;
right.bound = calc_bound(items, right, capacity);
if (right.bound > max_profit) {
Q.push(right);
}
}
return max_profit;
}
int main() {
vector<Item> items = {{10, 60}, {20, 100}, {30, 120}};
int capacity = 50;
int max_profit = knapsack(items, capacity);
cout << "Max Profit: " << max_profit << endl;
return 0;
}
```
在这个代码中,我们定义了一个`Item`结构体来存储物品的重量和价值信息,还定义了一个`Node`结构体来存储搜索状态空间中的结点信息。在`Node`结构体中,我们还重载了小于号运算符,这是为了让我们可以使用STL的最小堆来维护搜索结点的优先级。
在`calc_bound`函数中,我们计算了当前结点的价值上界,这是通过贪心的思路来计算的。具体来说,我们首先将当前结点的背包填满,然后尝试将下一个物品放入背包中,直到背包装不下为止。最后,我们将装不下的部分按照单位重量的价值比例来进行计算,从而得到当前结点的价值上界。
在`knapsack`函数中,我们使用了一个最小堆来维护搜索结点的优先级。在每一次循环中,我们取出最小堆中的顶部结点,然后根据当前结点的状态进行分支限界搜索。具体来说,我们分别考虑选择当前物品和不选择当前物品两种情况,然后计算子结点的价值上界,并将符合条件的子结点压入最小堆中。如果当前结点的价值上界小于已搜索到的最大价值,则可以剪枝,继续搜索下一个结点。
最后,在`main`函数中,我们定义了一个简单的0-1背包问题实例,然后调用`knapsack`函数求解,最终输出结果。
希望这个解答能够帮助到您!
阅读全文
相关推荐
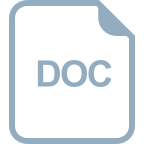
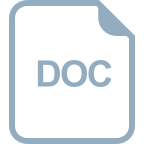
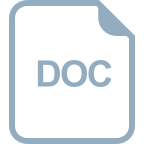
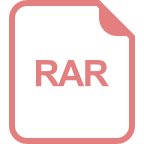
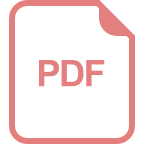
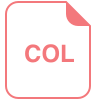
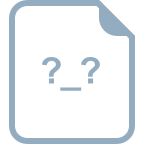
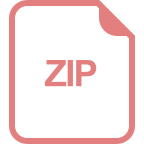
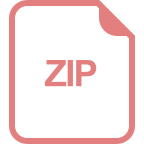
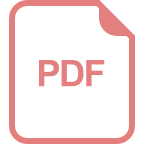
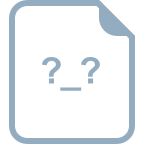
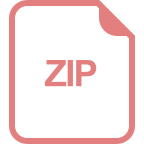
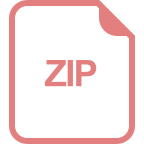
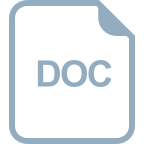
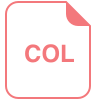
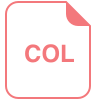
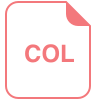
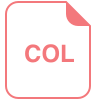