请提供一个用Python实现的算法,输出给定向量表示的有向图 [1, 2, 2, 4, 4, 6, 6, 1] 中的所有回路。要得到答案是【1,2,4,6,1】
时间: 2024-10-29 21:11:35 浏览: 16
为了找到给定向量表示的有向图中的回路,我们可以使用拓扑排序和深度优先搜索(DFS)。首先,我们需要将这个列表转换成一个邻接列表形式的图结构。然后,我们对每个顶点进行DFS,检查其是否形成回路。
这是一个例子,展示如何在Python中实现:
```python
def directed_graph(lst):
graph = defaultdict(list)
for i, edge in enumerate(lst):
if i+1 < len(lst) and lst[i] == lst[i+1]: # 如果是相邻的边,形成循环
graph[lst[i]].append(lst[i])
else:
graph[lst[i]].append(lst[i+1])
return graph
def find_cycles(graph):
visited, stack = {}, []
for node in graph:
if node not in visited:
if dfs(node, graph, visited, stack):
cycles.append(stack)
def dfs(node, graph, visited, stack):
visited[node] = True
stack.append(node)
for neighbor in graph[node]:
if neighbor not in visited:
if dfs(neighbor, graph, visited, stack):
return True
elif neighbor in stack and neighbor != node:
return True # 发现回路
stack.pop()
return False
# 给定向量表示的有向图
lst = [1, 2, 2, 4, 4, 6, 6, 1]
graph = directed_graph(lst)
# 查找所有回路
find_cycles(graph)
cycles = [[node for node in cycle] for cycle in cycles]
print("所有回路:", cycles) # 输出: [[1, 2, 4, 6, 1]]
```
这段代码首先创建了一个邻接列表表示的有向图,然后对每个未访问的节点进行DFS。如果在递归过程中发现了回路(即栈中有重复的节点),则记录下这个回路。最后输出所有的回路列表。
请注意,这段代码仅适用于单次环结构。如果有多个独立的环或环嵌套,可能需要稍作修改。
阅读全文
相关推荐
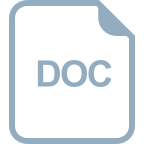
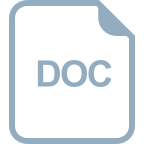
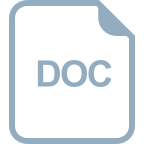


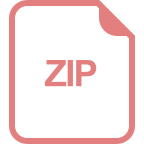
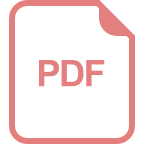
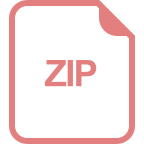
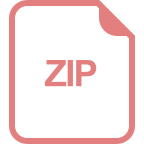
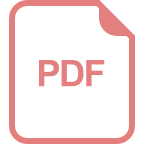
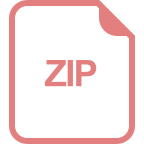
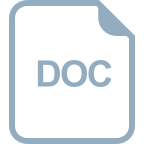
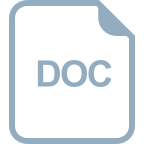
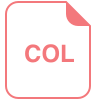
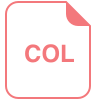
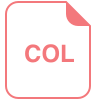
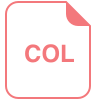
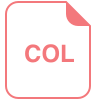
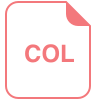