Qregexp exactMatch函数 替代
时间: 2024-01-02 08:02:56 浏览: 86
如果你需要在不使用 QRegExp 的情况下判断一个字符串是否完全匹配一个正则表达式,可以使用 C++11 引入的 std::regex 库。该库提供了 std::regex_match() 函数,可以实现类似 QRegExp::exactMatch() 的功能。以下是 std::regex_match() 函数的使用示例:
```cpp
#include <regex>
#include <iostream>
int main()
{
std::string str = "hello world";
std::regex regExp("hello");
if (std::regex_match(str, regExp)) {
std::cout << "Match!" << std::endl;
} else {
std::cout << "Not match!" << std::endl;
}
return 0;
}
```
在上述代码中,我们首先定义了一个字符串 str 和一个正则表达式 regExp。然后,我们使用 std::regex_match() 函数判断字符串 str 是否完全匹配正则表达式 regExp。根据正则表达式 "hello",字符串 "hello world" 是完全匹配的,因此该程序输出 "Match!"。需要注意的是,使用 std::regex_match() 函数时需要包含头文件 `<regex>`。
相关问题
Qregexp exactMatch函数
QRegExp::exactMatch() 是 Qt 框架提供的一个函数,用于判断一个字符串是否完全匹配一个正则表达式。该函数返回一个布尔值,如果字符串完全匹配正则表达式,返回 true,否则返回 false。以下是该函数的使用示例:
```cpp
#include <QRegExp>
#include <QDebug>
int main()
{
QString str = "hello world";
QRegExp regExp("hello");
if (regExp.exactMatch(str)) {
qDebug() << "Match!";
} else {
qDebug() << "Not match!";
}
return 0;
}
```
在上述代码中,我们首先定义了一个字符串 str 和一个正则表达式 regExp。然后,我们使用 QRegExp::exactMatch() 函数判断字符串 str 是否完全匹配正则表达式 regExp。根据正则表达式 "hello",字符串 "hello world" 是完全匹配的,因此该程序输出 "Match!"。
python QRegExp()函数功能及源代码
很抱歉,QRegExp()函数是Qt框架中的一个函数,与Python无关。它是用于在字符串中进行正则表达式匹配的函数,其功能与Python中的re模块类似。以下是一个示例代码:
```cpp
#include <QRegExp>
#include <QString>
#include <QDebug>
int main()
{
QString str = "Hello, world!";
// 创建正则表达式对象,匹配以“H”开头的单词
QRegExp regExp("^H\\w*");
// 判断字符串是否匹配正则表达式
if (regExp.exactMatch(str)) {
qDebug() << "Matched!";
} else {
qDebug() << "Not matched!";
}
return 0;
}
```
运行结果为:
```
Matched!
```
其中,`QRegExp("^H\\w*")`表示匹配以“H”开头的单词,`^`表示匹配字符串开头,`\w*`表示匹配零个或多个字母、数字或下划线。`exactMatch()`函数用于判断字符串是否完全匹配正则表达式。
阅读全文
相关推荐
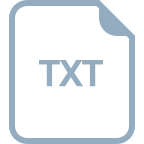
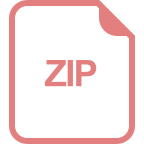
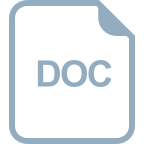











