QRegExp regex("([-+*/])");干啥的? QRegExp regex()这个函数是啥?
时间: 2024-04-17 13:28:32 浏览: 137
`QRegExp` 是一个用于处理正则表达式的类,它在Qt中被使用。`QRegExp regex("([-+*/])")` 这行代码定义了一个`QRegExp`对象 `regex`,并传入一个正则表达式作为参数。
在这个特定的正则表达式中,我们使用了括号 `()` 来表示一个捕获组,括号内的 `[-+*/]` 表示一个字符类,表示可以匹配 `+`、`-`、`*` 或 `/` 中的任意一个字符。这个正则表达式用于在表达式文本中查找到这些操作符。
在计算器的例子中,我们使用这个正则表达式来将表达式文本按照操作符进行分割,以便进行相应的计算操作。具体来说,我们使用 `split()` 函数将表达式文本根据操作符进行分割,分成数字和操作符两个部分,分别存储在 `numbers` 和 `operators` 字符串列表中。
关于 `QRegExp` 类的更多信息,您可以参考Qt官方文档:https://doc.qt.io/qt-5/qregexp.html
希望能够帮助您理解 `QRegExp regex("([-+*/])")` 这行代码的作用!如果还有其他问题,请随时提问。
相关问题
void MainWindow::on_buttonEqual_clicked() { QString expression = lineEditResult->text(); QRegExp regex("([-+*/])"); QStringList numbers = expression.split(regex, QString::SkipEmptyParts); QStringList operators = expression.split(QRegExp("\\d+"), QString::SkipEmptyParts); double result = numbers.at(0).toDouble(); for (int i = 1; i < numbers.size(); i++) { if (operators.at(i - 1) == "+") { result += numbers.at(i).toDouble(); } else if (operators.at(i - 1) == "-") { result -= numbers.at(i).toDouble(); } else if (operators.at(i - 1) == "*") { result *= numbers.at(i).toDouble(); } else if (operators.at(i - 1) == "/") { result /= numbers.at(i).toDouble(); } } lineEditResult->setText(QString::number(result)); }
这段代码是用于处理等号按钮的点击事件的槽函数。它会解析表达式并执行相应的计算操作,并将结果显示在结果文本框中。
具体的代码解释如下:
```cpp
void MainWindow::on_buttonEqual_clicked()
{
// 获取表达式文本
QString expression = lineEditResult->text();
// 使用正则表达式分割数字和操作符
QRegExp regex("([-+*/])");
QStringList numbers = expression.split(regex, QString::SkipEmptyParts);
QStringList operators = expression.split(QRegExp("\\d+"), QString::SkipEmptyParts);
// 执行计算操作
double result = numbers.at(0).toDouble();
for (int i = 1; i < numbers.size(); i++) {
if (operators.at(i - 1) == "+") {
result += numbers.at(i).toDouble();
} else if (operators.at(i - 1) == "-") {
result -= numbers.at(i).toDouble();
} else if (operators.at(i - 1) == "*") {
result *= numbers.at(i).toDouble();
} else if (operators.at(i - 1) == "/") {
result /= numbers.at(i).toDouble();
}
}
// 将计算结果显示在结果文本框中
lineEditResult->setText(QString::number(result));
}
```
在这段代码中,我们首先获取表达式文本通过 `lineEditResult->text()`。然后,使用正则表达式 `([-+*/])` 将数字和操作符分割成两个字符串列表,分别是 `numbers` 和 `operators`。
接下来,我们定义一个变量 `result` 用于保存计算结果,初始值为第一个数字。
然后,使用循环对每个操作符进行判断,根据不同的操作符进行相应的计算操作,然后将结果保存到 `result` 中。
最后,使用 `QString::number()` 将 `result` 转换为字符串,并将结果设置为结果文本框的文本。
这样,当等号按钮被点击时,就会触发 `on_buttonEqual_clicked()` 槽函数进行计算操作。
希望以上解释对您有所帮助!如果还有其他问题,请随时提问。
Qregexp exactMatch函数 替代
如果你需要在不使用 QRegExp 的情况下判断一个字符串是否完全匹配一个正则表达式,可以使用 C++11 引入的 std::regex 库。该库提供了 std::regex_match() 函数,可以实现类似 QRegExp::exactMatch() 的功能。以下是 std::regex_match() 函数的使用示例:
```cpp
#include <regex>
#include <iostream>
int main()
{
std::string str = "hello world";
std::regex regExp("hello");
if (std::regex_match(str, regExp)) {
std::cout << "Match!" << std::endl;
} else {
std::cout << "Not match!" << std::endl;
}
return 0;
}
```
在上述代码中,我们首先定义了一个字符串 str 和一个正则表达式 regExp。然后,我们使用 std::regex_match() 函数判断字符串 str 是否完全匹配正则表达式 regExp。根据正则表达式 "hello",字符串 "hello world" 是完全匹配的,因此该程序输出 "Match!"。需要注意的是,使用 std::regex_match() 函数时需要包含头文件 `<regex>`。
阅读全文
相关推荐
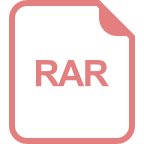
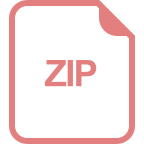
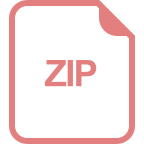













