用QT实现,将所有的G代码的格式写到QregExp,并且遍历这个数组表达式,实现G代码的解析
时间: 2024-04-30 21:21:10 浏览: 105
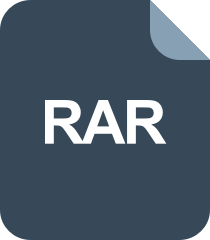
Qt使用QRegExp实现正则表达式处理
好的,那我会为你提供一个简单的实现步骤:
1. 创建一个QStringList类型的数组,用来存储所有的G代码格式。例如,可以这样定义:
```
QStringList gcode_format = {
"^G0( |\\t)*(X|Y|Z|E)*(-?\\d+\\.?\\d*)(X|Y|Z|E)*(-?\\d+\\.?\\d*)(X|Y|Z|E)*(-?\\d+\\.?\\d*)(F(-?\\d+\\.?\\d*))?$",
"^G1( |\\t)*(X|Y|Z|E)*(-?\\d+\\.?\\d*)(X|Y|Z|E)*(-?\\d+\\.?\\d*)(X|Y|Z|E)*(-?\\d+\\.?\\d*)(F(-?\\d+\\.?\\d*))?$",
...
};
```
2. 创建一个QRegExp类型的正则表达式,用来匹配G代码格式。例如,可以这样定义:
```
QRegExp gcode_regex;
```
3. 遍历gcode_format数组,将每个元素设置到正则表达式中,并且使用setPattern()方法进行设置。例如:
```
for (QString format : gcode_format) {
gcode_regex.setPattern(format);
// ...
}
```
4. 接下来,你需要读取G代码文件,并且使用正则表达式进行匹配。可以使用QFile和QTextStream来读取文件,并且使用QRegExp::indexIn()方法进行匹配。例如:
```
QFile file("gcode_file.gcode");
if (!file.open(QIODevice::ReadOnly | QIODevice::Text))
return;
QTextStream in(&file);
QString line;
while (!in.atEnd()) {
line = in.readLine();
int pos = gcode_regex.indexIn(line);
if (pos != -1) {
// 匹配成功,这里可以解析G代码参数
// ...
}
}
```
5. 在匹配成功后,你需要解析G代码参数。这里可以使用QRegExp::capturedTexts()方法来获取匹配到的子串,然后使用正则表达式来提取参数。例如:
```
QStringList captured = gcode_regex.capturedTexts();
QString command = captured[0];
QString x_value = captured[3];
QString y_value = captured[5];
QString z_value = captured[7];
QString e_value = captured[9];
QString f_value = captured[11];
```
6. 最后,你需要根据参数执行相应的操作。例如,可以将参数传递给一个函数,然后根据不同的G代码执行不同的操作。例如:
```
void execute_gcode(QString command, QString x_value, QString y_value, QString z_value, QString e_value, QString f_value) {
if (command == "G0") {
// G0操作
} else if (command == "G1") {
// G1操作
} else {
// 其他操作
}
}
```
阅读全文
相关推荐
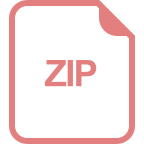
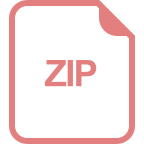
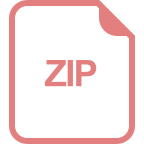
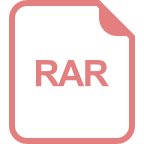
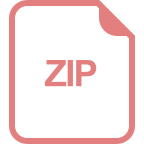
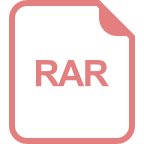
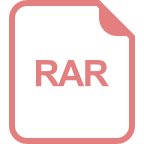
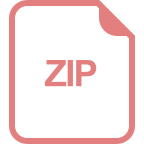
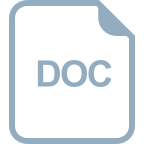





