请详细说明如何使用C语言完成一个有序单链表的创建、插入、合并以及遍历输出的具体步骤,并提供相应的代码实现。
时间: 2024-11-28 19:34:56 浏览: 3
要使用C语言实现单链表的相关操作,首先需要了解单链表的基本结构和操作原理。单链表由一系列节点组成,每个节点包含数据和指向下一个节点的指针。以下是创建、插入、合并以及遍历输出有序单链表的具体步骤和代码实现:
参考资源链接:[数据结构--单链表操作实验报告](https://wenku.csdn.net/doc/64658def543f844488aa9592?spm=1055.2569.3001.10343)
1. 创建单链表:
创建单链表需要定义节点结构体和链表头指针。节点结构体通常包含数据域和指向下一个节点的指针。
```c
typedef struct Node {
int data;
struct Node *next;
} Node;
Node* createList() {
Node *head = NULL, *tail = NULL;
int data;
while (scanf(
参考资源链接:[数据结构--单链表操作实验报告](https://wenku.csdn.net/doc/64658def543f844488aa9592?spm=1055.2569.3001.10343)
相关问题
如何使用C语言实现单链表的创建、插入、合并以及遍历输出,以完成一个有序单链表的合并操作?
在《数据结构--单链表操作实验报告》中,我们可以找到关于单链表操作的全面指导和演示,该资源将帮助你掌握从创建链表到执行复杂操作的整个流程。为了回答你的问题,以下是使用C语言实现单链表操作的具体步骤和代码示例:
参考资源链接:[数据结构--单链表操作实验报告](https://wenku.csdn.net/doc/64658def543f844488aa9592?spm=1055.2569.3001.10343)
1. 定义单链表节点结构体:
```c
typedef struct Node {
int data;
struct Node *next;
} Node;
```
2. 创建链表节点并插入数据:
```c
Node* createNode(int data) {
Node *newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
void insertInOrder(Node **head, int data) {
Node *current = *head;
Node *previous = NULL;
Node *newNode = createNode(data);
while (current != NULL && current->data < data) {
previous = current;
current = current->next;
}
if (previous == NULL) { // 插入到链表头部
newNode->next = *head;
*head = newNode;
} else { // 插入到链表中间或尾部
previous->next = newNode;
newNode->next = current;
}
}
```
3. 合并两个有序单链表:
```c
Node* mergeSortedLists(Node *head1, Node *head2) {
Node dummy;
Node *tail = &dummy;
dummy.next = NULL;
while (head1 && head2) {
if (head1->data <= head2->data) {
tail->next = head1;
head1 = head1->next;
} else {
tail->next = head2;
head2 = head2->next;
}
tail = tail->next;
}
tail->next = (head1 != NULL) ? head1 : head2;
return dummy.next;
}
```
4. 遍历输出单链表:
```c
void printList(Node *head) {
Node *current = head;
while (current != NULL) {
printf(
参考资源链接:[数据结构--单链表操作实验报告](https://wenku.csdn.net/doc/64658def543f844488aa9592?spm=1055.2569.3001.10343)
用C语言实现:两个有序单链表的合并到新的链表
可以按照以下步骤来实现两个有序单链表的合并到新的链表:
1. 定义链表节点结构体,包含数据和指向下一个节点的指针。
```c
struct ListNode {
int val;
struct ListNode *next;
};
```
2. 实现一个函数 `mergeTwoLists`,接收两个链表的头指针,返回合并后的链表头指针。
```c
struct ListNode* mergeTwoLists(struct ListNode* l1, struct ListNode* l2) {
// 如果两个链表中有一个为空,则直接返回另一个链表
if (l1 == NULL) return l2;
if (l2 == NULL) return l1;
// 定义一个新链表的头指针和尾指针
struct ListNode dummy;
struct ListNode *tail = &dummy;
// 遍历两个链表,将较小值的节点插入到新链表的尾部
while (l1 != NULL && l2 != NULL) {
if (l1->val < l2->val) {
tail->next = l1;
l1 = l1->next;
} else {
tail->next = l2;
l2 = l2->next;
}
tail = tail->next;
}
// 将剩余的节点插入到新链表尾部
if (l1 != NULL) tail->next = l1;
if (l2 != NULL) tail->next = l2;
// 返回新链表的头指针
return dummy.next;
}
```
这个函数会遍历两个链表,将较小值的节点插入到新链表的尾部,最后返回新链表的头指针。
可以使用以下代码测试这个函数的正确性:
```c
int main() {
struct ListNode l1_3 = {4, NULL};
struct ListNode l1_2 = {2, &l1_3};
struct ListNode l1_1 = {1, &l1_2};
struct ListNode l2_3 = {7, NULL};
struct ListNode l2_2 = {3, &l2_3};
struct ListNode l2_1 = {1, &l2_2};
struct ListNode *merged = mergeTwoLists(&l1_1, &l2_1);
while (merged != NULL) {
printf("%d ", merged->val);
merged = merged->next;
}
printf("\n");
return 0;
}
```
输出应该为:`1 1 2 3 4 7`。
阅读全文
相关推荐
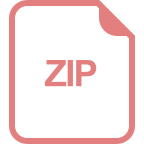
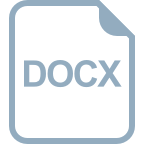
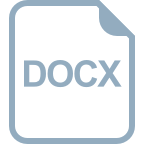





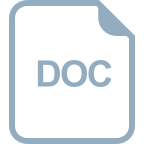
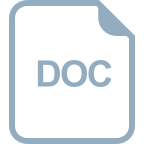






