pytorch之中x.t_()的结果是什么,用代码举例子详细说明
时间: 2023-04-05 18:04:09 浏览: 67
x.t_()是pytorch中的一个函数,它的作用是将张量x进行转置操作,并且将结果保存在原始张量x中。具体来说,x.t_()的返回值是None,但是x本身已经被转置了。
下面是一个示例代码,展示了x.t_()的使用方法:
```
import torch
# 创建一个2x3的张量
x = torch.tensor([[1, 2, 3], [4, 5, 6]])
# 执行转置操作
x.t_()
# 输出转置后的结果
print(x)
```
运行上述代码,输出结果如下:
```
tensor([[1, 4],
[2, 5],
[3, 6]])
```
可以看到,原始张量x被转置成了一个3x2的张量,并且结果保存在了x中。
相关问题
pytorch之中nn.parameter是什么,举例子用代码详细说明
nn.Parameter是一个类,它是一个可训练的参数张量。在PyTorch中,我们可以使用它来定义需要优化的参数。下面是一个例子:
```
import torch.nn as nn
class MyModel(nn.Module):
def __init__(self):
super(MyModel, self).__init__()
self.weight = nn.Parameter(torch.randn(3, 5))
self.bias = nn.Parameter(torch.zeros(3))
def forward(self, x):
output = torch.matmul(x, self.weight.t()) + self.bias
return output
```
在这个例子中,我们定义了一个MyModel类,它有两个参数:weight和bias。这两个参数都是nn.Parameter类型的张量。我们可以使用torch.randn()和torch.zeros()函数来初始化它们的值。在forward()函数中,我们使用weight和bias来计算输出。这些参数可以被优化器优化,以便模型可以更好地拟合数据。
pytorch之中requires_grad=True是什么意思?用代码详细说明
requires_grad=True是PyTorch中的一个参数,用于指定一个张量是否需要计算梯度。当requires_grad=True时,PyTorch会自动追踪该张量的计算历史,并在反向传播时计算该张量的梯度。
以下是一个示例代码,说明如何使用requires_grad=True:
```python
import torch
# 创建一个张量,并指定requires_grad=True
x = torch.tensor([1.0, 2.0, 3.0], requires_grad=True)
# 对张量进行计算
y = x * 2 + 1
# 对y进行求和
z = y.sum()
# 反向传播,计算x的梯度
z.backward()
# 输出x的梯度
print(x.grad)
```
在上面的代码中,我们创建了一个张量x,并指定requires_grad=True。然后对x进行计算,得到y。接着对y进行求和,得到z。最后调用z.backward(),PyTorch会自动计算z对x的梯度,并将结果保存在x.grad中。
相关推荐
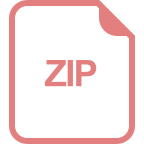













