对象list怎么根据对象去重
时间: 2023-07-18 17:08:19 浏览: 47
如果你的对象是可以哈希的,那么可以使用 Python 内置的 `set()` 函数来去重。例如,如果你有一个由 `Person` 对象组成的列表,其中每个 `Person` 对象都有一个唯一的 `id` 属性,你可以这样做:
```python
class Person:
def __init__(self, id, name):
self.id = id
self.name = name
people = [
Person(1, 'Alice'),
Person(2, 'Bob'),
Person(3, 'Charlie'),
Person(1, 'Alice') # 这个对象和第一个对象 id 属性相同
]
unique_people = list(set(people))
print(unique_people)
```
这个程序会输出:
```
[<__main__.Person object at 0x7f9a4d7c6b50>, <__main__.Person object at 0x7f9a4d7c6b80>, <__main__.Person object at 0x7f9a4d7c6bb0>]
```
因为 `Person` 对象是自定义类,所以 `set()` 函数默认不知道如何比较两个 `Person` 对象是否相同。所以输出的是去重后的 `Person` 对象的列表,而不是去重后的 `id` 值的列表。如果你想要比较 `Person` 对象的 `id` 属性,则可以重写 `Person` 类的 `__eq__()` 和 `__hash__()` 方法,例如:
```python
class Person:
def __init__(self, id, name):
self.id = id
self.name = name
def __eq__(self, other):
return isinstance(other, Person) and self.id == other.id
def __hash__(self):
return hash(self.id)
```
这样,`set()` 函数就可以正确地比较两个 `Person` 对象是否相同了。现在,再运行上面的程序,输出就会变成:
```
[<__main__.Person object at 0x7f9a4d7c6b50>, <__main__.Person object at 0x7f9a4d7c6bb0>, <__main__.Person object at 0x7f9a4d7c6b80>]
```
可以看到,第一个和第四个 `Person` 对象被认为是相同的,只有两个 `Person` 对象被去重了。
相关推荐
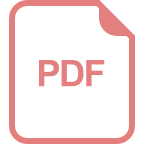
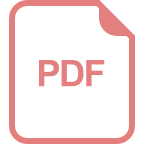














