用链表构建职工管理系统
时间: 2023-07-11 12:04:44 浏览: 101
职工管理系统可以使用链表来实现。链表是一种数据结构,它由一些列节点组成,每个节点包含一个数据元素和一个指向下一个节点的指针。
在职工管理系统中,每个职工可以看作一个节点,每个节点包含该职工的信息和一个指向下一个职工的指针。可以使用单向链表、双向链表或循环链表来实现。
下面是一个使用单向链表实现职工管理系统的示例代码:
```c++
#include <iostream>
using namespace std;
// 定义职工类
class Employee {
public:
int id; // 职工编号
string name; // 姓名
int age; // 年龄
int salary; // 工资
};
// 定义链表节点类
class Node {
public:
Employee emp; // 职工信息
Node* next; // 指向下一个节点的指针
};
// 定义链表类
class LinkedList {
public:
LinkedList() : head(NULL) {}
~LinkedList();
void insert(Employee emp); // 插入节点
void remove(int id); // 删除节点
void display(); // 显示链表中所有节点
private:
Node* head; // 链表头指针
};
LinkedList::~LinkedList() {
Node* current = head;
while (current != NULL) {
Node* temp = current;
current = current->next;
delete temp;
}
}
void LinkedList::insert(Employee emp) {
Node* newNode = new Node;
newNode->emp = emp;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
} else {
Node* current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
void LinkedList::remove(int id) {
if (head == NULL) {
return;
}
if (head->emp.id == id) {
Node* temp = head;
head = head->next;
delete temp;
return;
}
Node* current = head;
while (current->next != NULL) {
if (current->next->emp.id == id) {
Node* temp = current->next;
current->next = temp->next;
delete temp;
return;
}
current = current->next;
}
}
void LinkedList::display() {
Node* current = head;
while (current != NULL) {
cout << "ID: " << current->emp.id << endl;
cout << "Name: " << current->emp.name << endl;
cout << "Age: " << current->emp.age << endl;
cout << "Salary: " << current->emp.salary << endl;
cout << endl;
current = current->next;
}
}
int main() {
LinkedList list;
// 添加职工
Employee emp1 = { 1, "Amy", 25, 3000 };
list.insert(emp1);
Employee emp2 = { 2, "Bob", 30, 4000 };
list.insert(emp2);
Employee emp3 = { 3, "Charlie", 35, 5000 };
list.insert(emp3);
// 显示职工列表
list.display();
// 删除职工
list.remove(2);
// 再次显示职工列表
list.display();
return 0;
}
```
在这个示例中,我们定义了一个职工类 Employee,其中包含职工的编号、姓名、年龄和工资等信息。我们还定义了一个链表节点类 Node,其中包含一个职工对象和一个指向下一个节点的指针。
在链表类 LinkedList 中,我们定义了插入节点、删除节点和显示链表中所有节点的方法。其中,插入节点方法是将一个新节点插入到链表的末尾;删除节点方法是根据职工编号删除指定节点;显示链表中所有节点方法是遍历链表并输出每个节点的职工信息。
在主函数中,我们创建了三个职工对象并插入到链表中,然后显示链表中所有节点,并删除了编号为 2 的职工节点,最后再次显示链表中所有节点。
阅读全文
相关推荐
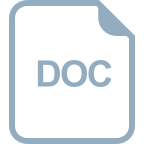


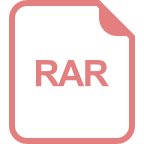
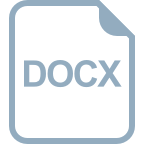
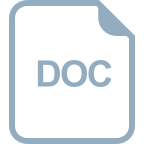
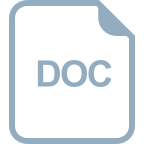
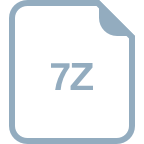
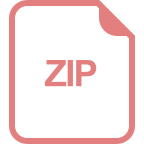
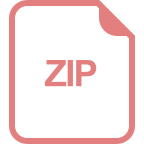
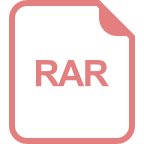
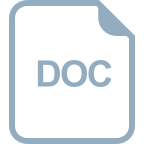
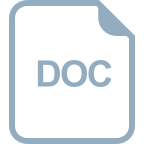
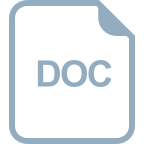
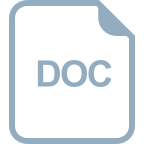
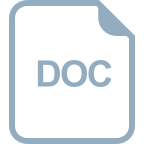