Reed-Solomon coding in programming languages such as python
时间: 2023-09-16 13:15:28 浏览: 53
Reed-Solomon coding is widely used in error correcting codes, particularly in data storage and transmission applications. In Python, you can implement Reed-Solomon coding using libraries such as NumPy and Py-ECC. Py-ECC provides fast and optimized Reed-Solomon encoding and decoding functions. Another popular library is FEC, which provides a pure Python implementation of Reed-Solomon codes. You can also find many tutorials and code examples online to help you get started with implementing Reed-Solomon coding in Python.
相关问题
reed-solomon:关于如何在python中实现
Reed-Solomon是一种具有错误纠正能力的编码技术,常被用于数据传输和存储中。
在Python中,我们可以使用一些已有的库来实现Reed-Solomon编码。其中最常用的是`python-rs-codec`库。首先,你需要安装该库,可以通过`pip install rs-codec`命令进行安装。
下面是一个使用`python-rs-codec`库实现Reed-Solomon编码的简单示例:
```python
from rs_codec import RSCodec
def encode_reed_solomon(data, n, k):
rs = RSCodec(n - k) # 创建一个Reed-Solomon编码器
# 对数据进行编码
encoded_data = rs.encode(data)
return encoded_data
def decode_reed_solomon(encoded_data, n, k):
rs = RSCodec(n - k) # 创建一个Reed-Solomon编码器
# 对编码后的数据进行解码
decoded_data = rs.decode(encoded_data)
return decoded_data
# 测试
data = bytearray(b"Hello World")
n = 10 # 编码后的数据长度
k = 5 # 原始数据长度(无冗余数据)
encoded_data = encode_reed_solomon(data, n, k)
decoded_data = decode_reed_solomon(encoded_data, n, k)
print("原始数据:", data)
print("编码后的数据:", encoded_data)
print("解码后的数据:", decoded_data)
```
上述示例代码中,`encode_reed_solomon`函数用于对数据进行编码,传入参数为原始数据、编码后数据的总长度n和原始数据的长度k。`decode_reed_solomon`函数用于对编码后的数据进行解码,传入参数同样为编码后数据的总长度n和原始数据的长度k。
这样,我们就可以在Python中使用`python-rs-codec`库实现Reed-Solomon编码和解码的功能了。请确保在使用示例代码前正确安装了该库。
reed-solomon-fec
Reed-Solomon前向纠错(Forward Error Correction,FEC)是一种在数据传输过程中用来纠正错误的技术。它被广泛应用在数字通信、数据存储和网络传输等领域。
Reed-Solomon FEC通过在数据中增加冗余信息来提高数据传输的可靠性。在发送端,原始数据会被编码成多个数据块,并且每个数据块都会包含一些冗余信息。这些冗余信息可以帮助接收端在数据发生错误时进行纠正,并恢复原始数据。
与其他纠错编码技术相比,Reed-Solomon FEC拥有很高的纠错能力,甚至可以容忍大量的数据错误。这使得它特别适用于那些对数据传输可靠性要求很高的场景。
另外,Reed-Solomon FEC也具有比较高的编码和解码效率,这意味着它可以在不增加太多传输延迟的情况下提供很好的纠错功能。
总的来说,Reed-Solomon FEC是一种可靠高效的前向纠错技术,它对于保障数据传输的完整性和可靠性起着非常重要的作用。在实际应用中,它可以帮助我们在面对各种数据传输问题时,更好地保护数据的完整性。
相关推荐
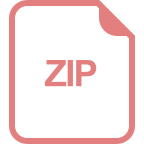
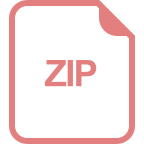
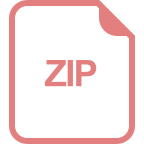












