C++ opencv SURF 图像拼接
时间: 2023-10-08 22:08:27 浏览: 158
在C++中使用OpenCV进行SURF图像拼接的步骤如下:
1. 导入必要的头文件和命名空间:
```
#include <opencv2/opencv.hpp>
#include <opencv2/xfeatures2d.hpp>
using namespace cv;
using namespace cv::xfeatures2d;
```
2. 加载要拼接的图片:
```
Mat img1 = imread("image1.jpg");
Mat img2 = imread("image2.jpg");
```
3. 提取SURF特征点:
```
std::vector<KeyPoint> keyPoint1, keyPoint2;
Ptr<Feature2D> surfFeature = xfeatures2d::SURF::create(2000); // 设置SURF参数
surfFeature->detect(img1, keyPoint1);
surfFeature->detect(img2, keyPoint2);
```
4. 计算特征描述子:
```
Mat desc1, desc2;
surfFeature->compute(img1, keyPoint1, desc1);
surfFeature->compute(img2, keyPoint2, desc2);
```
5. 匹配特征点:
```
Ptr<cv::DescriptorMatcher> matcher = cv::DescriptorMatcher::create(cv::DescriptorMatcher::FLANNBASED);
std::vector<cv::DMatch> matches;
matcher->match(desc1, desc2, matches);
```
6. 选择最佳匹配的特征点:
```
double minDist = 100;
for (int i = 0; i < desc1.rows; i++) {
double dist = matches[i].distance;
if (dist < minDist) {
minDist = dist;
}
}
std::vector<cv::DMatch> goodMatches;
for (int i = 0; i < desc1.rows; i++) {
if (matches[i].distance <= std::max(2 * minDist, 0.02)) {
goodMatches.push_back(matches[i]);
}
}
```
7. 寻找图像的变换矩阵:
```
std::vector<cv::Point2f> srcPoints;
std::vector<cv::Point2f> dstPoints;
for (int i = 0; i < goodMatches.size(); i++) {
srcPoints.push_back(keyPoint1[goodMatches[i].queryIdx].pt);
dstPoints.push_back(keyPoint2[goodMatches[i].trainIdx].pt);
}
cv::Mat homography = cv::findHomography(srcPoints, dstPoints, cv::RANSAC);
```
8. 拼接图像:
```
cv::Mat stitchedImg;
cv::warpPerspective(img1, stitchedImg, homography, cv::Size(img1.cols + img2.cols, img1.rows));
cv::Mat roi(stitchedImg, cv::Rect(0, 0, img2.cols, img2.rows));
img2.copyTo(roi);
```
9. 显示结果:
```
cv::imshow("Stitched Image", stitchedImg);
cv::waitKey(0);
```
阅读全文
相关推荐
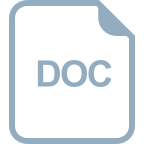
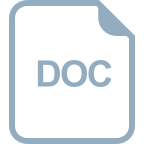
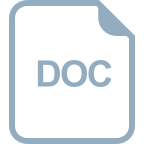

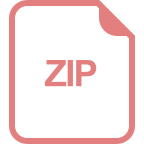
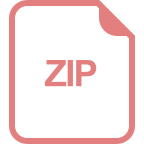





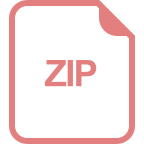
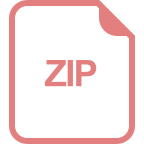
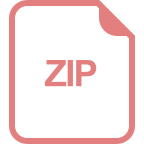
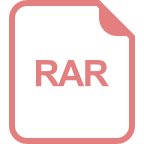
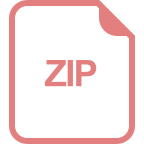
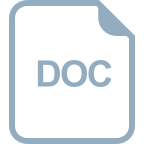
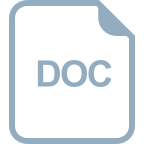
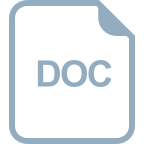