实现基于opencv的图像拼接c++代码,要求可以实现三张图片的拼接
时间: 2024-09-12 07:13:31 浏览: 23
在C++中使用OpenCV进行图像拼接,通常是通过特征匹配和变换矩阵计算来实现的。OpenCV提供了几个函数和类,比如`ORB`、`SIFT`、`SURF`等用于特征检测和匹配,以及`cv::findHomography`用于计算变换矩阵,`cv::warpPerspective`用于图像变换。以下是一个简单的示例代码,展示了如何使用OpenCV实现三张图片的拼接:
```cpp
#include <opencv2/opencv.hpp>
#include <vector>
using namespace cv;
using namespace std;
vector<Mat> images; // 存储输入图像
vector<Mat> images_gray; // 存储灰度图像
vector<Mat> images_mask; // 存储掩码图像
// 加载图像并转换为灰度
void LoadImages() {
images.push_back(imread("image1.jpg"));
images.push_back(imread("image2.jpg"));
images.push_back(imread("image3.jpg"));
for(int i = 0; i < images.size(); i++) {
cvtColor(images[i], images_gray[i], COLOR_BGR2GRAY);
}
}
// 特征检测和匹配
void FeatureMatching() {
// 创建ORB检测器
Ptr<Feature2D> detector = ORB::create();
vector<DMatch> matches;
// 用于存储匹配结果的矩阵
Mat img_matches;
// 使用ORB检测特征点和提取描述子
for(int i = 0; i < images.size(); i++) {
vector<KeyPoint> keypoints;
detector->detect(images_gray[i], keypoints);
Mat descriptors;
detector->compute(images_gray[i], keypoints, descriptors);
// 特征匹配
for(int j = 0; j < images.size(); j++) {
vector<KeyPoint> keypoints2;
Mat descriptors2;
detector->detect(images_gray[j], keypoints2);
detector->compute(images_gray[j], keypoints2, descriptors2);
vector<DMatch> matches_temp;
BFMatcher matcher(NORM_HAMMING);
matcher.match(descriptors, descriptors2, matches_temp);
// 可以通过距离过滤出好的匹配点
// 这里简化处理,未进行过滤
img_matches.push_back(images[i]);
img_matches.push_back(images[j]);
}
}
// 显示匹配结果
// 这里只是示意,实际开发中可能需要更复杂的显示方式
imshow("Matches", img_matches);
waitKey(0);
}
int main() {
LoadImages();
FeatureMatching();
return 0;
}
```
上面的代码仅提供了一个基本的框架和思路,实际的图像拼接过程可能需要更复杂的处理,例如:
- 对每个图像使用特征检测器提取特征点和描述子。
- 使用特征匹配算法找出两个图像之间的匹配点。
- 通过匹配点计算单应性矩阵(Homography matrix)。
- 使用得到的变换矩阵对图像进行变换,然后进行图像融合。
- 图像融合的处理可能需要使用掩码和混合技术以平滑接缝。
请注意,由于拼接过程涉及到图像变换和融合,可能还需要考虑图像重叠区域的选择、融合权重的计算等复杂因素。
相关推荐
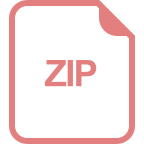
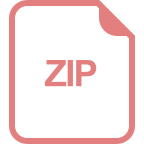
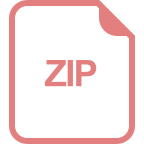














