编写程序对明文进行哈夫曼编码
时间: 2024-12-10 16:46:23 浏览: 21
哈夫曼编码是一种用于数据压缩的统计编码方法,特别适合于字符频度较高的文本。以下是使用Python编写一个简单的哈夫曼树及其编码过程的基本步骤:
1. **计算频率**:首先统计输入文本中每个字符出现的频率,通常存储在一个字典中,键是字符,值是对应的频率。
```python
from collections import Counter
text = "Hello, World!"
char_freq = Counter(text)
```
2. **构建哈夫曼树**:利用哈夫曼树构造算法(也称为赫夫曼树),将频率高的节点排在前面,并不断合并两个最轻的节点直到只剩下一个,这个过程可以使用优先队列(例如`heapq`模块的`heappush`和`heappop`函数)来高效地操作。
```python
import heapq
def build_huffman_tree(freqs):
heap = [[weight, [char, '']] for char, weight in freqs.items()]
heapq.heapify(heap)
while len(heap) > 1:
left = heapq.heappop(heap)
right = heapq.heappop(heap)
new_node = [left[0] + right[0], []]
new_node[1].append(left[1])
new_node[1].append(right[1])
heapq.heappush(heap, new_node)
return heap[0][1]
huffman_tree = build_huffman_tree(char_freq)
```
3. **生成编码规则**:从根节点开始,对于每个叶子节点(即字符),记录下从根到该叶节点路径上所有边的“0”、“1”序列,这就是该字符的哈夫曼编码。
```python
def get_code(node, path=''):
if not node[1]:
return path
else:
return get_code(node[1][0], path + '0') + get_code(node[1][1], path + '1')
huffman_codes = {char: get_code(node) for char, node in zip(huffman_tree, char_freq.keys())}
```
4. **应用编码**:最后,你可以遍历原始文本,替换每个字符为其哈夫曼编码,得到压缩后的字符串。
```python
compressed_text = ''.join([huffman_codes[char] for char in text])
```
阅读全文
相关推荐
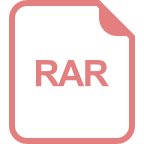
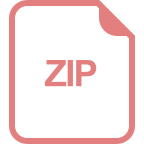
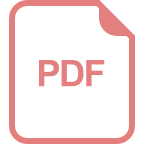
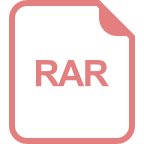
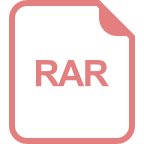
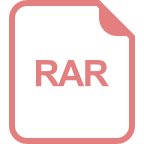
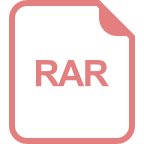
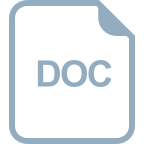
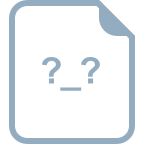
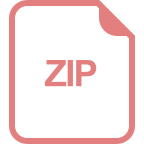
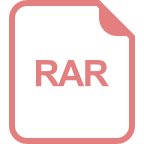
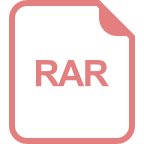
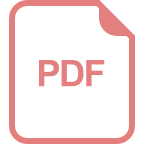
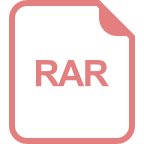
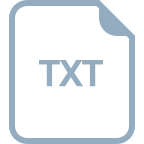
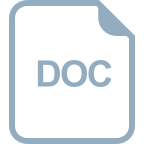