讲解一下matlab中的ischar
时间: 2024-01-06 16:03:52 浏览: 174
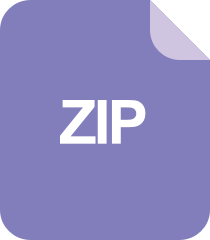
matlab中文字符的识别代码
`ischar` 是 MATLAB 内置函数之一,用于检查一个变量是否为字符数组(字符串)类型。其语法为:
```
tf = ischar(A)
```
其中,`A` 为待检查的变量,`tf` 返回一个布尔值,表示 `A` 是否为字符数组。如果 `A` 是字符数组,则返回值为 true,否则返回 false。
例如,以下代码检查一个变量 `str` 是否为字符数组类型:
```
str = 'Hello, world!';
if ischar(str)
disp('str is a character array.')
else
disp('str is not a character array.')
end
```
如果 `str` 是字符数组类型,则输出 `str is a character array.`。如果 `str` 不是字符数组,则输出 `str is not a character array.`。
`ischar` 函数在 MATLAB 中广泛应用,常用于判断用户输入是否为字符串类型、判断文件名是否为字符串类型等等。
阅读全文
相关推荐
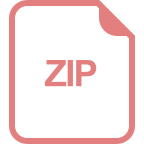
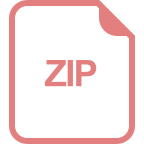
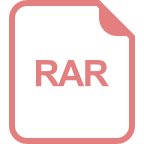
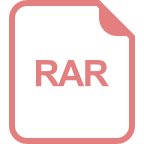
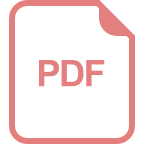
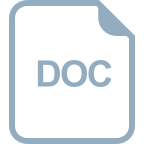
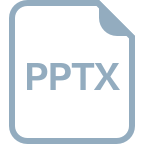
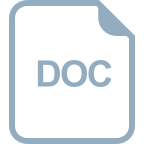
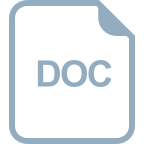
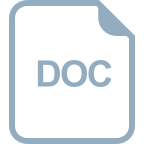
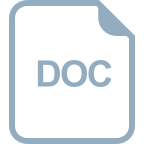
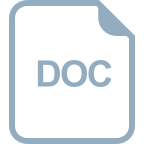
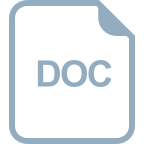
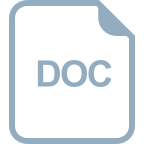
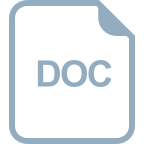
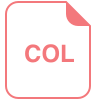
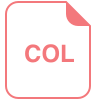
