用skimage实现答题卡的识别
时间: 2023-12-05 07:04:19 浏览: 37
要使用skimage实现答题卡的识别,可以按照以下步骤操作:
1. 导入skimage模块和其他需要的模块,如numpy和matplotlib。
2. 读取答题卡的图像,可以使用skimage.io模块的imread函数。
3. 对图像进行预处理,包括灰度化、二值化、边缘检测等操作,以便更好地分离出答题卡和答案区域。
4. 使用skimage.measure模块的find_contours函数找到答案区域的轮廓。
5. 对轮廓进行处理,包括去除不必要的轮廓、按照顺序排列轮廓等操作。
6. 根据轮廓的位置和形状确定答案区域的位置和大小。
7. 将答案区域进行分割,得到每个选项的图像。
8. 对每个选项的图像进行处理,包括二值化、去除噪声等操作,以便更好地识别。
9. 使用OCR技术对每个选项的图像进行识别,得到对应的答案。
10. 根据识别结果确定最终的答案。
以上是使用skimage实现答题卡的识别的基本步骤,具体实现时需要根据具体情况进行调整和优化。
相关问题
用skimage实现答题卡识别系统的代码及原理
答题卡识别系统是一种基于图像处理技术实现的自动化识别答题卡的系统。下面是用skimage实现答题卡识别系统的步骤和代码:
1. 图像预处理:使用skimage库中的函数将原始图像转换为灰度图像,并进行二值化处理。
```
import skimage.io as io
import skimage.color as color
import skimage.filters as filters
# 读取原始图像
image = io.imread('test.png')
# 转换为灰度图像
gray_image = color.rgb2gray(image)
# 二值化处理
threshold_value = filters.threshold_otsu(gray_image)
binary_image = gray_image > threshold_value
```
2. 轮廓提取:使用skimage库中的函数提取二值化图像的轮廓信息。
```
import skimage.measure as measure
# 提取轮廓信息
contours = measure.find_contours(binary_image, 0.8)
```
3. 答题卡定位:根据轮廓信息确定答题卡的位置和大小。
```
# 初始化答题卡位置和大小
min_x = binary_image.shape[1]
max_x = 0
min_y = binary_image.shape[0]
max_y = 0
# 遍历所有轮廓
for contour in contours:
# 计算轮廓的最小外接矩形
min_row, min_col = np.min(contour, axis=0)
max_row, max_col = np.max(contour, axis=0)
if min_row < min_y:
min_y = min_row
if max_row > max_y:
max_y = max_row
if min_col < min_x:
min_x = min_col
if max_col > max_x:
max_x = max_col
# 答题卡位置和大小
card_x = min_x
card_y = min_y
card_width = max_x - min_x
card_height = max_y - min_y
```
4. 答案识别:根据答题卡的位置和大小,提取出答案区域的图像,并进行识别。
```
# 提取答案区域的图像
answer_image = binary_image[card_y+card_height//2:card_y+card_height, card_x:card_x+card_width]
# 答案识别
answer = []
for i in range(num_questions):
x1 = int(i * card_width / num_questions)
x2 = int((i + 1) * card_width / num_questions)
answer_area = answer_image[:, x1:x2]
if np.sum(answer_area) > answer_threshold:
answer.append(1)
else:
answer.append(0)
```
以上就是用skimage实现答题卡识别系统的步骤和代码。
用skimage实现答题卡的识别和判断
要使用skimage实现答题卡的识别和判断,可以按照以下步骤进行:
1. 首先使用skimage读入答题卡的图像,可以使用以下代码:
```python
from skimage import io
image = io.imread('answer_sheet.jpg')
```
2. 对图像进行预处理,包括灰度化、二值化、去噪等操作,可以使用以下代码:
```python
from skimage import color, filters
gray_image = color.rgb2gray(image)
binary_image = gray_image > filters.threshold_otsu(gray_image)
denoised_image = filters.median(binary_image)
```
3. 使用skimage中的Hough变换来检测出答题卡的边缘,可以使用以下代码:
```python
from skimage.transform import probabilistic_hough_line
lines = probabilistic_hough_line(denoised_image, threshold=10, line_length=5, line_gap=3)
```
4. 根据检测到的边缘,将答题卡从原图中分割出来,可以使用以下代码:
```python
import numpy as np
from skimage.draw import polygon
xmin = np.min([line[0][0] for line in lines])
ymin = np.min([line[0][1] for line in lines])
xmax = np.max([line[0][0] for line in lines])
ymax = np.max([line[0][1] for line in lines])
mask = np.zeros_like(gray_image)
rr, cc = polygon([ymin, ymax, ymax, ymin], [xmin, xmin, xmax, xmax])
mask[rr, cc] = 1
answer_sheet = np.multiply(gray_image, mask)
```
5. 对答题卡中的选项区域进行检测和识别,可以使用一些机器学习或深度学习的方法来训练模型,识别出选项区域,并判断每个选项是否被选中。
以上就是使用skimage实现答题卡的识别和判断的基本步骤。当然,具体实现还需要根据具体的场景和需求进行调整和完善。
相关推荐
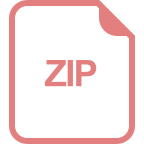
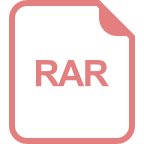
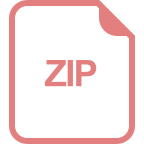












