实现链栈的建立进栈出栈和返回栈顶元素输出算法
时间: 2024-05-03 21:20:58 浏览: 14
好的,可以的。链栈的建立可以使用链表的方式实现,进栈可以在链表头插入节点,出栈可以在链表头删除节点,返回栈顶元素可以直接返回链表头节点的值。下面是基于链表实现的链栈算法:
1. 定义链栈节点结构体:
```c
typedef struct node {
int data;
struct node *next;
} Node;
```
2. 初始化链栈:
```c
Node *top = NULL; // 链栈顶指针
```
3. 进栈算法:
```c
void push(int value) { // value 是要进栈的值
Node *new_node = (Node *)malloc(sizeof(Node));
if (new_node == NULL) {
printf("内存分配失败\n");
return;
}
new_node->data = value;
new_node->next = top;
top = new_node;
}
```
4. 出栈算法:
```c
void pop() {
if (top == NULL) {
printf("链栈已经为空,无法出栈\n");
return;
}
Node *temp = top;
top = top->next;
free(temp);
}
```
5. 返回栈顶元素算法:
```c
int top_value() {
if (top == NULL) {
printf("链栈为空,无法返回栈顶元素\n");
return -1; // 假设栈中不可能存在 -1
}
return top->data;
}
```
希望以上算法可以帮助您实现链栈。接下来请问您有什么其他问题吗?
相关推荐
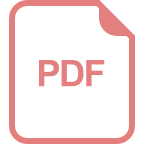







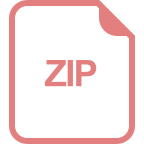
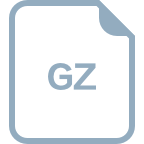
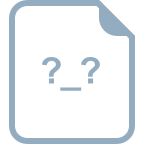