c++写出时间片轮转调度算法
时间: 2023-06-12 13:07:06 浏览: 172
时间片轮转调度算法是一种常见的 CPU 调度算法,它将 CPU 时间分为若干个时间片(通常为几十毫秒),每个进程被分配一个时间片,当时间片用完后,该进程被挂起,等待下一次轮转到它时再继续执行。以下是使用 C 语言实现时间片轮转调度算法的示例代码:
#include <stdio.h>
struct Process {
int pid; // 进程 ID
int burst_time; // 进程需要的 CPU 时间
int remaining_time; // 剩余的 CPU 时间
};
void round_robin(struct Process processes[], int n, int time_quantum) {
int current_time = 0;
int total_waiting_time = 0;
int total_turnaround_time = 0;
int completed_processes = 0;
// 循环执行进程直到所有进程完成
while (completed_processes < n) {
for (int i = 0; i < n; i++) {
// 如果进程已经完成,则跳过
if (processes[i].remaining_time == 0) {
continue;
}
// 执行进程,并更新进程剩余时间
int executed_time = processes[i].remaining_time > time_quantum ? time_quantum : processes[i].remaining_time;
processes[i].remaining_time -= executed_time;
// 更新当前时间和进程的等待时间
current_time += executed_time;
total_waiting_time += current_time - (processes[i].burst_time - processes[i].remaining_time);
// 如果进程已经完成,更新进程的周转时间
if (processes[i].remaining_time == 0) {
total_turnaround_time += current_time - processes[i].burst_time;
completed_processes++;
}
}
}
// 输出结果
float average_waiting_time = (float)total_waiting_time / n;
float average_turnaround_time = (float)total_turnaround_time / n;
printf("Average waiting time: %.2f\n", average_waiting_time);
printf("Average turnaround time: %.2f\n", average_turnaround_time);
}
int main() {
struct Process processes[] = {
{1, 10, 10},
{2, 5, 5},
{3, 8, 8},
{4, 4, 4},
{5, 3, 3}
};
int n = sizeof(processes) / sizeof(processes[0]);
int time_quantum = 2;
round_robin(processes, n, time_quantum);
return 0;
}
在该示例中,我们定义了一个 Process
结构体来表示进程,并实现了一个 round_robin
函数来执行时间片轮转调度算法。在 round_robin
函数中,我们使用一个循环来依次执行每个进程,如果进程已经完成,则跳过;否则,执行该进程,并更新进程的剩余时间、当前时间和进程的等待时间。如果进程已经完成,则更新进程的周转时间并将已完成进程计数器加一。最后,我们输出平均等待时间和平均周转时间。在 main
函数中,我们定义了一个进程数组,并调用 round_robin
函数来执行时间片轮转调度算法。
相关推荐



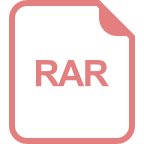














