const targetDate = new Date('2023-04-24'); const now = new Date(); const timeDiff = targetDate.getTime() - now.getTime();这段代码是什么意思
时间: 2024-05-28 21:14:28 浏览: 23
这段代码计算了距离目标日期`2023-04-24`还有多少时间差,单位是毫秒。首先,通过`new Date()`创建了当前时间的`Date`对象,然后通过`getTime()`方法获取到当前时间的时间戳;接着通过`new Date('2023-04-24')`创建了目标日期的`Date`对象,同样通过`getTime()`方法获取到目标日期的时间戳;最后用目标日期的时间戳减去当前时间的时间戳,得到的时间差即为距离目标日期的毫秒数,保存在变量`timeDiff`中。
相关问题
用JS写现在距离2023年4月24日过了多少年月多少日多少小时多少分多少秒
可以使用JavaScript的Date对象来完成这个任务。
首先,我们需要创建一个表示2023年4月24日的Date对象:
```javascript
const targetDate = new Date('2023-04-24');
```
接着,我们可以获取当前时间的Date对象:
```javascript
const now = new Date();
```
然后,我们可以计算两个时间之间的毫秒数差:
```javascript
const timeDiff = targetDate.getTime() - now.getTime();
```
接下来,我们可以将毫秒数转换为年、月、日、小时、分钟和秒:
```javascript
const seconds = Math.floor(timeDiff / 1000);
const minutes = Math.floor(seconds / 60);
const hours = Math.floor(minutes / 60);
const days = Math.floor(hours / 24);
const months = Math.floor(days / 30.44);
const years = Math.floor(months / 12);
```
最后,我们可以输出结果:
```javascript
console.log(`距离2023年4月24日过了${years}年${months % 12}月${days % 30.44}天${hours % 24}小时${minutes % 60}分${seconds % 60}秒`);
```
完整代码如下:
```javascript
const targetDate = new Date('2023-04-24');
const now = new Date();
const timeDiff = targetDate.getTime() - now.getTime();
const seconds = Math.floor(timeDiff / 1000);
const minutes = Math.floor(seconds / 60);
const hours = Math.floor(minutes / 60);
const days = Math.floor(hours / 24);
const months = Math.floor(days / 30.44);
const years = Math.floor(months / 12);
console.log(`距离2023年4月24日过了${years}年${months % 12}月${days % 30.44}天${hours % 24}小时${minutes % 60}分${seconds % 60}秒`);
```
为了掌握定时器和Date对象创建一个倒计时页面利用倒计时,每100毫秒变化一次倒计时
好的,我可以为您提供一些代码参考,帮助您实现这个倒计时页面。具体实现步骤如下:
1. 在 HTML 页面上创建一个显示倒计时的元素,比如一个 div 标签。
2. 在 JavaScript 中使用 Date 对象获取当前时间和目标时间,并计算出两者之间的时间差。
3. 使用 setInterval 方法每隔 100 毫秒更新倒计时页面,直到时间差为 0。
下面是参考代码:
HTML 页面:
```html
<div id="countdown"></div>
```
JavaScript 代码:
```javascript
// 获取目标时间,这里假设目标时间为 2022 年 1 月 1 日 0 时 0 分 0 秒
const targetDate = new Date('2022-01-01T00:00:00');
// 更新倒计时页面的函数
function updateCountdown() {
// 获取当前时间
const currentDate = new Date();
// 计算时间差
const timeDiff = targetDate.getTime() - currentDate.getTime();
// 如果时间差小于等于 0,停止倒计时
if (timeDiff <= 0) {
clearInterval(intervalId);
document.getElementById('countdown').innerHTML = '倒计时结束!';
return;
}
// 将时间差转换为天、小时、分钟、秒和毫秒
let days = Math.floor(timeDiff / (1000 * 60 * 60 * 24));
let hours = Math.floor((timeDiff % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
let minutes = Math.floor((timeDiff % (1000 * 60 * 60)) / (1000 * 60));
let seconds = Math.floor((timeDiff % (1000 * 60)) / 1000);
let milliseconds = timeDiff % 1000;
// 更新倒计时页面
document.getElementById('countdown').innerHTML = `倒计时:${days}天 ${hours}小时 ${minutes}分 ${seconds}秒 ${milliseconds}毫秒`;
}
// 每隔 100 毫秒更新倒计时页面
const intervalId = setInterval(updateCountdown, 100);
```
这段代码会在页面上显示一个倒计时,每隔 100 毫秒更新一次,直到目标时间为止。您可以根据自己的需要修改目标时间和页面元素的 ID。
相关推荐
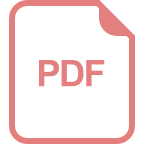
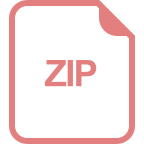
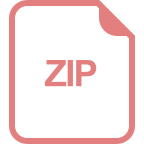








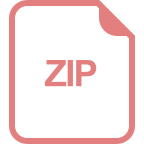
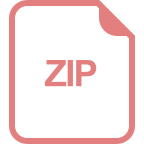
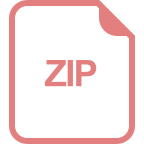
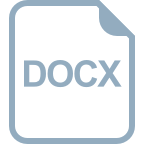
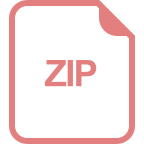