vue3 ts 右键菜单
时间: 2023-09-10 18:10:51 浏览: 162
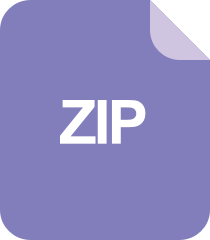
右键菜单
可以使用 Vue3 和 TypeScript 来创建一个右键菜单组件,可以按照以下步骤进行操作:
1. 创建一个新的 Vue3 组件,并在其中定义一个 data 对象,用于存储右键菜单的状态:
```typescript
<script lang="ts">
import { defineComponent } from 'vue';
export default defineComponent({
data() {
return {
menuVisible: false,
menuX: 0,
menuY: 0,
};
},
});
</script>
```
2. 在组件的 template 中,定义一个 div 元素,并将其绑定到右键事件上:
```typescript
<template>
<div @contextmenu.prevent="showMenu" />
</template>
```
3. 实现 showMenu 方法,用于显示右键菜单。该方法需要接收一个 MouseEvent 对象,以获取鼠标点击的位置:
```typescript
<script lang="ts">
import { defineComponent } from 'vue';
export default defineComponent({
data() {
return {
menuVisible: false,
menuX: 0,
menuY: 0,
};
},
methods: {
showMenu(event: MouseEvent) {
this.menuVisible = true;
this.menuX = event.pageX;
this.menuY = event.pageY;
},
},
});
</script>
```
4. 在 template 中,根据 menuVisible 来决定是否显示右键菜单:
```typescript
<template>
<div @contextmenu.prevent="showMenu" />
<div
v-show="menuVisible"
class="context-menu"
:style="{ left: menuX + 'px', top: menuY + 'px' }"
>
<!-- 右键菜单内容 -->
</div>
</template>
```
5. 在 CSS 中,为右键菜单添加样式:
```css
.context-menu {
position: absolute;
background-color: white;
border: 1px solid #ccc;
padding: 5px;
z-index: 9999;
}
```
现在,你已经创建了一个简单的右键菜单组件,可以在其中添加更多的菜单选项和交互逻辑,以满足你的需求。
阅读全文
相关推荐

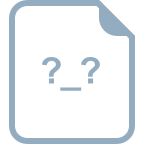
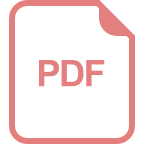
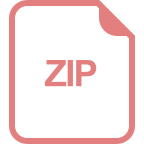
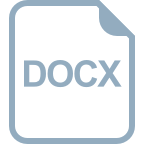
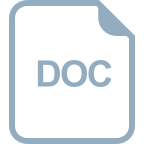






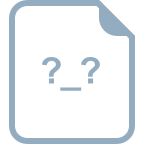
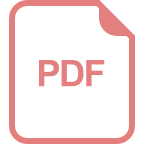

