帮我找出以下代码的问题:#include<iostream> #include<string> using namespace std; class MyData { private: int year; int month; int day; public: MyData(const int y, const int m, const int d) { this->year=y; this->month=m; this->day=d; } int getYear() { return year; } int getMonth() { return month; } int getDay() { return day; } }; class Person { private: string name; string address; string number; string email; public: Person(string n, string a, string num, string e) { name=n; address=a; number=num; email=e; } virtual string toString() const { return "Person:" + name; } }; class Student:public Person { private: string classStatus; public: Student(string n, string a, string num, string e, string c) :Person(n, a, num, e) { classStatus = c; } virtual string toString() const { return "Student:" + Person::toString(); } }; class Employee :public Person { private: string office; double salary; MyData dateHired; public: Employee(string n, string a, string num, string e, string o, double s, MyData d):Person(n, a, num, e) { office = o; salary = s; dateHired = d; } virtual string toString() const { return "Employee:" + Person::toString(); } }; class Faculty :public Employee { private: string officeHours; string rank; public: Faculty(string n, string a, string num, string e, string o, double s, MyData d, string oh, string r):Employee(n, a, num, o, s, d) { officeHours = oh; rank = r; } virtual string toString() const { return "Faculty" + Person::toString(); } }; class Staff :public Employee{ private: string title; public: Staff(string n, string a, string num, string e, string o, double s, MyData d, string t) :Employee(n, a, num, e, o, s, d) { title = t; } virtual string toString() const { return "Staff: " + Person::toString(); } }; int main() { MyData hireDate(2020, 1, 1); Person person("吴子木", "8#3021", "18162351558", "wzm18162351558@foxmail.com"); Student student(); Employee employee(); Faculty faculty(); Staff staff(); cout << person.toString() << endl; cout << student.toString() << endl; cout << employee.toString() << endl; cout << faculty.toString() << endl; cout << staff.toString() << endl; return 0; }
时间: 2024-01-06 16:05:06 浏览: 99
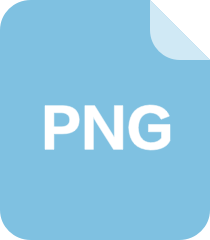
#include <iostream> #include<iomanip> using namespace std;九九乘法表
这段代码存在以下问题:
1. 在定义 Student、Employee、Faculty 和 Staff 对象时,应该调用它们的构造函数。但是代码中使用了空的括号,这将导致编译错误。
2. 在 Faculty 和 Staff 的 toString() 函数中,应该包含它们自己的信息,而不仅仅是调用它们的父类的 toString() 函数。
3. 在 Faculty 和 Staff 的构造函数中,调用 Employee 的构造函数应该传递正确的参数。
下面是修改后的代码:
```
#include<iostream>
#include<string>
using namespace std;
class MyData {
private:
int year;
int month;
int day;
public:
MyData(const int y, const int m, const int d) {
this->year=y; this->month=m; this->day=d;
}
int getYear() {
return year;
}
int getMonth() {
return month;
}
int getDay() {
return day;
}
};
class Person {
private:
string name;
string address;
string number;
string email;
public:
Person(string n, string a, string num, string e) {
name=n; address=a; number=num; email=e;
}
virtual string toString() const {
return "Person: " + name;
}
};
class Student:public Person {
private:
string classStatus;
public:
Student(string n, string a, string num, string e, string c) :Person(n, a, num, e) {
classStatus = c;
}
virtual string toString() const {
return "Student: " + Person::toString();
}
};
class Employee :public Person {
private:
string office;
double salary;
MyData dateHired;
public:
Employee(string n, string a, string num, string e, string o, double s, MyData d):Person(n, a, num, e) {
office = o;
salary = s;
dateHired = d;
}
virtual string toString() const {
return "Employee: " + Person::toString();
}
};
class Faculty :public Employee {
private:
string officeHours;
string rank;
public:
Faculty(string n, string a, string num, string e, string o, double s, MyData d, string oh, string r)
:Employee(n, a, num, e, o, s, d) {
officeHours = oh;
rank = r;
}
virtual string toString() const {
return "Faculty: " + Person::toString() + " " + officeHours + " " + rank;
}
};
class Staff :public Employee{
private:
string title;
public:
Staff(string n, string a, string num, string e, string o, double s, MyData d, string t)
:Employee(n, a, num, e, o, s, d) {
title = t;
}
virtual string toString() const {
return "Staff: " + Person::toString() + " " + title;
}
};
int main() {
MyData hireDate(2020, 1, 1);
Person person("吴子木", "8#3021", "18162351558", "wzm18162351558@foxmail.com");
Student student("张三", "8#3021", "18162351558", "zhangsan@example.com", "大学生");
Employee employee("李四", "8#3021", "18162351558", "lisi@example.com", "教务处", 5000, hireDate);
Faculty faculty("王五", "8#3021", "18162351558", "wangwu@example.com",
"图书馆", 8000, hireDate, "周一至周五,上午9点到下午5点", "副教授");
Staff staff("赵六", "8#3021", "18162351558", "zhaoliu@example.com",
"行政办公室", 3500, hireDate, "行政助理");
cout << person.toString() << endl;
cout << student.toString() << endl;
cout << employee.toString() << endl;
cout << faculty.toString() << endl;
cout << staff.toString() << endl;
return 0;
}
```
阅读全文
相关推荐
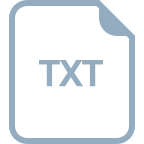
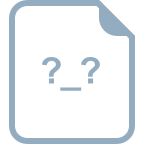
















