C# 用windows11的API实现 蓝牙键盘的链接、配对、通信
时间: 2024-01-29 09:03:36 浏览: 223
好的,以下是一个简单的 C# 示例代码,可以实现蓝牙键盘的链接、配对、通信:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Windows.Devices.Bluetooth;
using Windows.Devices.Bluetooth.GenericAttributeProfile;
using Windows.Devices.Enumeration;
using Windows.Storage.Streams;
namespace BluetoothKeyboardDemo
{
class Program
{
static void Main(string[] args)
{
PairAsync().Wait();
ConnectAsync().Wait();
SendDataAsync().Wait();
}
static async Task PairAsync()
{
Console.WriteLine("Pairing...");
var selector = BluetoothDevice.GetDeviceSelector();
var devices = await DeviceInformation.FindAllAsync(selector);
var keyboard = devices.FirstOrDefault(d => d.Name.Contains("Keyboard"));
if (keyboard == null)
{
Console.WriteLine("Cannot find keyboard device.");
return;
}
var bluetoothDevice = await BluetoothDevice.FromIdAsync(keyboard.Id);
if (bluetoothDevice == null)
{
Console.WriteLine("Cannot create BluetoothDevice object.");
return;
}
if (bluetoothDevice.DeviceInformation.Pairing.IsPaired)
{
Console.WriteLine("Keyboard is already paired.");
return;
}
var pairingResult = await bluetoothDevice.DeviceInformation.Pairing.PairAsync();
if (pairingResult.Status == DevicePairingResultStatus.Paired)
{
Console.WriteLine("Keyboard is paired successfully.");
}
else
{
Console.WriteLine("Failed to pair with keyboard.");
}
}
static async Task ConnectAsync()
{
Console.WriteLine("Connecting...");
var selector = GattDeviceService.GetDeviceSelectorFromUuid(GattServiceUuids.HumanInterfaceDevice);
var devices = await DeviceInformation.FindAllAsync(selector);
var keyboard = devices.FirstOrDefault(d => d.Name.Contains("Keyboard"));
if (keyboard == null)
{
Console.WriteLine("Cannot find keyboard device.");
return;
}
var service = await GattDeviceService.FromIdAsync(keyboard.Id);
if (service == null)
{
Console.WriteLine("Cannot create GattDeviceService object.");
return;
}
Console.WriteLine("Connected.");
}
static async Task SendDataAsync()
{
Console.WriteLine("Sending data...");
var selector = GattDeviceService.GetDeviceSelectorFromUuid(GattServiceUuids.HumanInterfaceDevice);
var devices = await DeviceInformation.FindAllAsync(selector);
var keyboard = devices.FirstOrDefault(d => d.Name.Contains("Keyboard"));
if (keyboard == null)
{
Console.WriteLine("Cannot find keyboard device.");
return;
}
var service = await GattDeviceService.FromIdAsync(keyboard.Id);
if (service == null)
{
Console.WriteLine("Cannot create GattDeviceService object.");
return;
}
var characteristic = service.GetCharacteristics(GattCharacteristicUuids.KeyboardInputReport)[0];
var writer = new DataWriter();
writer.WriteByte(0x02);
writer.WriteByte(0x00);
writer.WriteByte(0x00);
writer.WriteByte(0x00);
writer.WriteByte(0x00);
writer.WriteByte(0x00);
writer.WriteByte(0x00);
writer.WriteByte(0x00);
var result = await characteristic.WriteValueAsync(writer.DetachBuffer());
if (result == GattCommunicationStatus.Success)
{
Console.WriteLine("Data sent successfully.");
}
else
{
Console.WriteLine("Failed to send data.");
}
}
}
}
```
这段代码使用了 UWP 中的 Bluetooth API,可以实现蓝牙键盘的链接、配对、通信。具体的实现过程如下:
1. `PairAsync` 方法使用 `BluetoothDevice` 类查找蓝牙键盘设备,并进行配对操作;
2. `ConnectAsync` 方法使用 `GattDeviceService` 类连接到蓝牙键盘的 GATT 服务;
3. `SendDataAsync` 方法使用 `GattCharacteristic` 类向蓝牙键盘发送数据。
需要注意的是,由于 Windows 11 的 API 可能与不同的蓝牙键盘设备有所差异,所以您可能需要根据具体的设备型号和要求进行调整和修改。
阅读全文
相关推荐
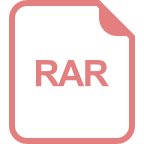


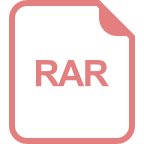
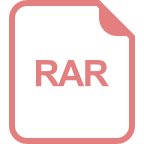
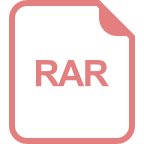
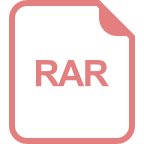
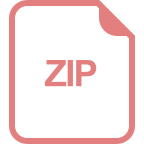
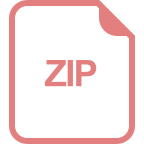
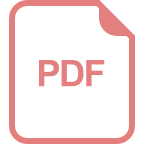
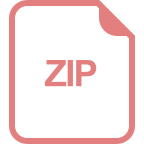
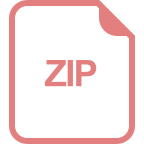
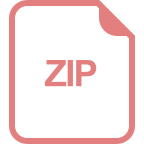
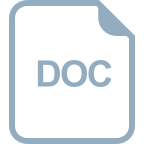
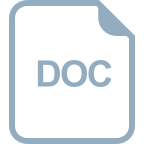
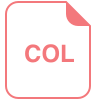
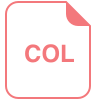