C# 用windows11 API 实现低功耗蓝牙蓝牙键盘的扫描、配对、通信例程
时间: 2023-12-24 18:05:45 浏览: 230
好的,我可以回答你的问题。首先,需要使用 C# 语言和 Windows 11 API 来实现低功耗蓝牙键盘的扫描、配对和通信。下面是一个简单的例程,可以帮助你开始。
首先,在你的项目中添加对 Windows.Devices.Bluetooth 命名空间的引用,以便使用 Windows 11 API。
接下来,你需要使用以下代码来扫描低功耗蓝牙设备:
```csharp
private async void ScanForDevices()
{
var watcher = DeviceInformation.CreateWatcher(
BluetoothLEDevice.GetDeviceSelectorFromPairingState(false),
null,
DeviceInformationKind.AssociationEndpoint);
watcher.Added += Watcher_Added;
watcher.Start();
}
private async void Watcher_Added(DeviceWatcher sender, DeviceInformation deviceInfo)
{
BluetoothLEDevice bluetoothLeDevice = await BluetoothLEDevice.FromIdAsync(deviceInfo.Id);
if (bluetoothLeDevice != null)
{
// 处理扫描到的蓝牙设备
}
}
```
在上面的代码中,我们创建了一个 DeviceWatcher 对象,并设置了 Added 事件处理程序。当扫描到设备时,Watcher_Added 方法将被调用,并获取 BluetoothLEDevice 对象以进行进一步处理。
接下来,你可以使用以下代码来配对和连接低功耗蓝牙设备:
```csharp
BluetoothLEDevice bluetoothLeDevice = await BluetoothLEDevice.FromIdAsync(deviceInfo.Id);
if (bluetoothLeDevice != null)
{
DevicePairingResult result = await bluetoothLeDevice.DeviceInformation.Pairing.PairAsync();
if (result.Status == DevicePairingResultStatus.Paired)
{
// 设备已成功配对
// 现在可以建立连接并开始通信
}
}
```
在上面的代码中,我们使用 FromIdAsync 方法获取 BluetoothLEDevice 对象,并使用 PairAsync 方法对设备进行配对。如果配对成功,我们可以使用 BluetoothLEDevice 对象建立连接并开始通信。
最后,你可以使用以下代码来发送和接收数据:
```csharp
GattDeviceService service = await bluetoothLeDevice.GetGattServicesForUuidAsync(serviceUuid);
if (service != null)
{
GattCharacteristic characteristic = await service.GetCharacteristicsForUuidAsync(characteristicUuid);
if (characteristic != null)
{
byte[] data = Encoding.UTF8.GetBytes("Hello world!");
await characteristic.WriteValueAsync(data.AsBuffer());
}
}
```
在上面的代码中,我们使用 GetGattServicesForUuidAsync 方法获取 GattDeviceService 对象,并使用 GetCharacteristicsForUuidAsync 方法获取 GattCharacteristic 对象。然后,我们可以使用 WriteValueAsync 方法将数据发送到设备,或使用 ReadValueAsync 方法从设备接收数据。
希望这个例程对你有帮助!
阅读全文
相关推荐
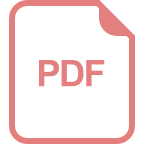





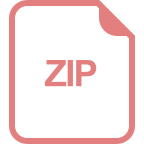

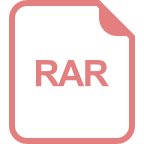
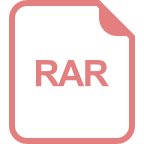
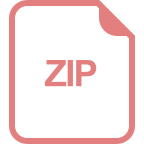
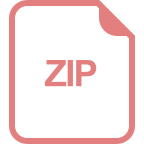
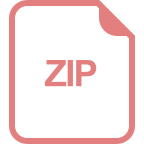
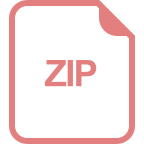
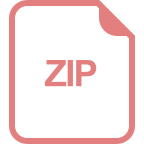
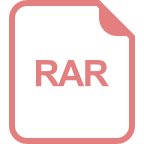
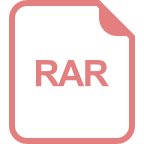