C# 用windows11 API 实现低功耗蓝牙的扫描、配对、通信例程
时间: 2023-12-24 11:05:07 浏览: 44
好的,我会尽力回答您的问题。首先,您需要了解一些关于 Windows 11 的 API 和 C# 语言的基础知识。接下来,我将分为三个部分来回答您的问题:低功耗蓝牙扫描、配对和通信。
1. 低功耗蓝牙扫描
要使用 Windows 11 API 进行低功耗蓝牙扫描,您需要使用 BluetoothLEAdvertisementWatcher 类。以下是一个简单的示例:
```
using System;
using Windows.Devices.Bluetooth;
using Windows.Devices.Bluetooth.Advertisement;
public class BluetoothScanner
{
private BluetoothLEAdvertisementWatcher watcher;
public BluetoothScanner()
{
watcher = new BluetoothLEAdvertisementWatcher();
watcher.ScanningMode = BluetoothLEScanningMode.Active;
watcher.Received += Watcher_Received;
}
public void Start()
{
watcher.Start();
}
public void Stop()
{
watcher.Stop();
}
private void Watcher_Received(BluetoothLEAdvertisementWatcher sender, BluetoothLEAdvertisementReceivedEventArgs args)
{
// 处理扫描到的蓝牙设备
}
}
```
在上面的示例中,我们创建了一个 BluetoothScanner 类来处理扫描。在构造函数中,我们实例化了 BluetoothLEAdvertisementWatcher 对象,并将扫描模式设置为 BluetoothLEScanningMode.Active。然后,我们订阅了 Received 事件,该事件在扫描到蓝牙设备时被触发。在事件处理程序中,我们可以处理扫描到的设备。
2. 低功耗蓝牙配对
要使用 Windows 11 API 进行低功耗蓝牙配对,您需要使用 BluetoothDevice 类。以下是一个简单的示例:
```
using System;
using Windows.Devices.Bluetooth;
using Windows.Devices.Enumeration;
public class BluetoothPairer
{
public async Task<BluetoothDevice> PairAsync(string deviceId)
{
var device = await BluetoothDevice.FromIdAsync(deviceId);
if (device.DeviceInformation.Pairing.CanPair)
{
var result = await device.DeviceInformation.Pairing.PairAsync();
if (result.Status == DevicePairingResultStatus.Paired)
{
return device;
}
else
{
// 处理配对失败的情况
}
}
else
{
// 处理设备无法配对的情况
}
return null;
}
}
```
在上面的示例中,我们创建了一个 BluetoothPairer 类来处理配对。在 PairAsync 方法中,我们传入设备的 Id,然后实例化 BluetoothDevice 对象。然后,我们检查设备是否可以配对,如果可以,我们调用 PairAsync 方法进行配对。最后,我们检查配对结果,并返回 BluetoothDevice 对象。
3. 低功耗蓝牙通信
要使用 Windows 11 API 进行低功耗蓝牙通信,您需要使用 GattCharacteristic 类。以下是一个简单的示例:
```
using System;
using System.Linq;
using System.Threading.Tasks;
using Windows.Devices.Bluetooth;
using Windows.Devices.Bluetooth.GenericAttributeProfile;
public class BluetoothCommunicator
{
public async Task<string> SendMessageAsync(BluetoothDevice device, string message)
{
var service = await GetServiceAsync(device);
var characteristic = await GetCharacteristicAsync(service);
var buffer = Windows.Security.Cryptography.CryptographicBuffer.ConvertStringToBinary(message, Windows.Security.Cryptography.BinaryStringEncoding.Utf8);
var result = await characteristic.WriteValueAsync(buffer);
if (result == GattCommunicationStatus.Success)
{
var readResult = await characteristic.ReadValueAsync();
var readBuffer = readResult.Value;
var response = Windows.Security.Cryptography.CryptographicBuffer.ConvertBinaryToString(Windows.Security.Cryptography.BinaryStringEncoding.Utf8, readBuffer);
return response;
}
else
{
// 处理写入失败的情况
}
return null;
}
private async Task<GattDeviceService> GetServiceAsync(BluetoothDevice device)
{
var services = await device.GetGattServicesAsync();
var service = services.Services.FirstOrDefault(s => s.Uuid == GattServiceUuids.GenericAccess);
if (service == null)
{
// 处理找不到服务的情况
}
return service;
}
private async Task<GattCharacteristic> GetCharacteristicAsync(GattDeviceService service)
{
var characteristics = await service.GetCharacteristicsAsync();
var characteristic = characteristics.Characteristics.FirstOrDefault(c => c.Uuid == GattCharacteristicUuids.DeviceName);
if (characteristic == null)
{
// 处理找不到特征的情况
}
return characteristic;
}
}
```
在上面的示例中,我们创建了一个 BluetoothCommunicator 类来处理通信。在 SendMessageAsync 方法中,我们传入 BluetoothDevice 对象和消息字符串。然后,我们获取 GattDeviceService 对象和 GattCharacteristic 对象,并将消息写入特征。最后,我们读取响应并返回响应字符串。
希望这些示例能够帮助您开始使用 Windows 11 API 进行低功耗蓝牙扫描、配对和通信。如果您有任何其他问题,请随时问我。
相关推荐
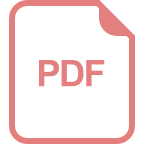
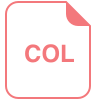













