C# 用windows11 API 实现经典蓝牙的扫描、配对、通信例程
时间: 2023-12-24 08:05:07 浏览: 35
好的,以下是一个简单的C#代码示例,用于使用Windows 11 API实现经典蓝牙的扫描、配对、通信:
```
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Windows.Devices.Bluetooth;
using Windows.Devices.Enumeration;
namespace BluetoothApp
{
class Program
{
static async Task Main(string[] args)
{
// 扫描蓝牙设备
var bluetoothDevices = await DeviceInformation.FindAllAsync(BluetoothDevice.GetDeviceSelector());
foreach (var device in bluetoothDevices)
{
// 显示设备名称和ID
Console.WriteLine($"Device Name: {device.Name}, Device ID: {device.Id}");
}
// 选择一个蓝牙设备进行配对
var selectedDevice = bluetoothDevices.FirstOrDefault();
if (selectedDevice != null)
{
var bluetoothDevice = await BluetoothDevice.FromIdAsync(selectedDevice.Id);
// 配对蓝牙设备
var result = await bluetoothDevice.Pairing.TryPinPairingAsync();
if (result.Status == BluetoothPairingResultStatus.Paired)
{
Console.WriteLine("Bluetooth device paired successfully!");
// 连接蓝牙设备
var connectionResult = await bluetoothDevice.GetRfcommServicesForIdAsync(BluetoothService.SerialPort);
var service = connectionResult.Services.FirstOrDefault();
var socket = new Windows.Networking.Sockets.StreamSocket();
await socket.ConnectAsync(service.ConnectionHostName, service.ConnectionServiceName);
Console.WriteLine("Bluetooth device connected successfully!");
// 发送和接收数据
var writer = new Windows.Storage.Streams.DataWriter(socket.OutputStream);
writer.WriteString("Hello, Bluetooth!");
var reader = new Windows.Storage.Streams.DataReader(socket.InputStream);
await reader.LoadAsync(512);
var message = reader.ReadString(reader.UnconsumedBufferLength);
Console.WriteLine($"Received message: {message}");
// 断开连接
socket.Dispose();
}
else
{
Console.WriteLine($"Failed to pair Bluetooth device, status: {result.Status}");
}
}
else
{
Console.WriteLine("No Bluetooth devices found!");
}
Console.ReadKey();
}
}
}
```
请注意,此代码示例仅用于演示目的。实际实现可能需要更复杂的逻辑和错误处理。
相关推荐
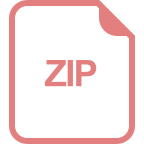














