3.分别把 string, list, tuple, dict类型的数据写入到文件中
时间: 2023-12-04 07:05:22 浏览: 34
写入string类型数据到文件:
```python
string_data = "Hello, world!"
with open("file.txt", "w") as f:
f.write(string_data)
```
写入list类型数据到文件:
```python
list_data = [1, 2, 3, 4, 5]
with open("file.txt", "w") as f:
for item in list_data:
f.write(str(item) + "\n")
```
写入tuple类型数据到文件:
```python
tuple_data = ("apple", "banana", "cherry")
with open("file.txt", "w") as f:
for item in tuple_data:
f.write(item + "\n")
```
写入dict类型数据到文件:
```python
dict_data = {"name": "Alice", "age": 25, "city": "New York"}
with open("file.txt", "w") as f:
for key, value in dict_data.items():
f.write(key + ": " + str(value) + "\n")
```
相关问题
python练习将 string, list, tuple, dict类型的数据写入到文件中
以下是将不同类型的数据写入文件的示例代码:
1. 写入字符串到文件
```python
text = "Hello, world!"
with open("file.txt", "w") as f:
f.write(text)
```
2. 写入列表到文件
```python
my_list = [1, 2, 3, 4, 5]
with open("file.txt", "w") as f:
for item in my_list:
f.write(str(item) + "\n")
```
3. 写入元组到文件
```python
my_tuple = (1, 2, 3, 4, 5)
with open("file.txt", "w") as f:
for item in my_tuple:
f.write(str(item) + "\n")
```
4. 写入字典到文件
```python
my_dict = {"name": "John", "age": 30, "city": "New York"}
with open("file.txt", "w") as f:
for key, value in my_dict.items():
f.write(key + ":" + str(value) + "\n")
```
注意:在写入数据时,要确保将所有数据转换为字符串类型,因为文件只能保存字符串类型的数据。
用python把 string, list, tuple, dict类型的数据写入到文件里
可以使用Python内置的文件操作函数来写入不同类型的数据到文件中。
1. 写入字符串类型的数据
```python
data = "Hello World\nWelcome to Python\n"
with open("data.txt", "w") as f:
f.write(data)
```
2. 写入列表类型的数据
```python
data = ["apple", "banana", "orange"]
with open("data.txt", "w") as f:
for item in data:
f.write("%s\n" % item)
```
3. 写入元组类型的数据
```python
data = ("apple", "banana", "orange")
with open("data.txt", "w") as f:
for item in data:
f.write("%s\n" % item)
```
4. 写入字典类型的数据
```python
data = {"apple": 2, "banana": 3, "orange": 4}
with open("data.txt", "w") as f:
for key, value in data.items():
f.write("%s:%s\n" % (key, value))
```
在这些示例中,我们使用了Python的with语句来打开文件,并将数据写入文件。在with块结束时,文件会自动关闭。
相关推荐
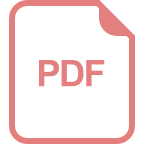
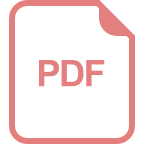
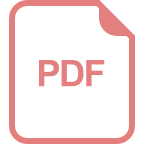












