python分别把string,list,tuple,dict写入文件中
时间: 2024-05-14 07:13:35 浏览: 178
可以使用Python内置的文件操作函数将string、list、tuple、dict写入文件中:
1. 将string写入文件
```python
text = "Hello, world!"
with open("file.txt", "w") as f:
f.write(text)
```
2. 将list写入文件
```python
mylist = [1, 2, 3, 4, 5]
with open("file.txt", "w") as f:
for i in mylist:
f.write(str(i) + "\n")
```
3. 将tuple写入文件
```python
mytuple = (1, 2, 3, 4, 5)
with open("file.txt", "w") as f:
for i in mytuple:
f.write(str(i) + "\n")
```
4. 将dict写入文件
```python
mydict = {"name": "Alice", "age": 25, "city": "New York"}
with open("file.txt", "w") as f:
for key, value in mydict.items():
f.write(key + ":" + str(value) + "\n")
```
以上代码都是将数据按行写入到文件中,如果需要其他格式,可以自行修改。
相关问题
用python编写,分别把string,list,tuple,dict写入文件中
Sure! 首先,我们需要先定义一个文件路径和名称,比如 "data.txt"。然后我们可以按照以下方式将这些数据类型写入文件中:
1. String 写入文件:
```
my_string = "Hello World!"
with open("data.txt", "w") as file:
file.write(my_string)
```
这会将字符串 "Hello World!" 写入名为 "data.txt" 的文件中。
2. List 写入文件:
```
my_list = [1, 2, 3, 4, 5]
with open("data.txt", "w") as file:
for item in my_list:
file.write(str(item) + "\n")
```
这会将列表 [1, 2, 3, 4, 5] 写入名为 "data.txt" 的文件中,在每个元素下面添加一个换行符。
3. Tuple 写入文件:
```
my_tuple = (1, 2, 3, 4, 5)
with open("data.txt", "w") as file:
for item in my_tuple:
file.write(str(item) + "\n")
```
这会将元组 (1, 2, 3, 4, 5) 写入名为 "data.txt" 的文件中,在每个元素下面添加一个换行符。
4. Dictionary 写入文件:
```
my_dict = {"name": "John Smith", "age": 25, "salary": 5000}
with open("data.txt", "w") as file:
for key, value in my_dict.items():
file.write(str(key) + ": " + str(value) + "\n")
```
这会将字典 {"name": "John Smith", "age": 25, "salary": 5000} 写入名为 "data.txt" 的文件中,每个键值对之间用冒号分隔。在每个键值对下面添加一个换行符。
用python把 string, list, tuple, dict类型的数据写入到文件里
可以使用Python内置的文件操作函数来写入不同类型的数据到文件中。
1. 写入字符串类型的数据
```python
data = "Hello World\nWelcome to Python\n"
with open("data.txt", "w") as f:
f.write(data)
```
2. 写入列表类型的数据
```python
data = ["apple", "banana", "orange"]
with open("data.txt", "w") as f:
for item in data:
f.write("%s\n" % item)
```
3. 写入元组类型的数据
```python
data = ("apple", "banana", "orange")
with open("data.txt", "w") as f:
for item in data:
f.write("%s\n" % item)
```
4. 写入字典类型的数据
```python
data = {"apple": 2, "banana": 3, "orange": 4}
with open("data.txt", "w") as f:
for key, value in data.items():
f.write("%s:%s\n" % (key, value))
```
在这些示例中,我们使用了Python的with语句来打开文件,并将数据写入文件。在with块结束时,文件会自动关闭。
阅读全文
相关推荐
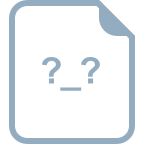
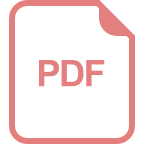
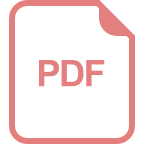




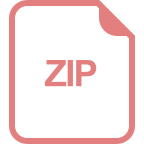
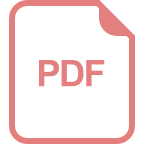
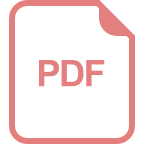
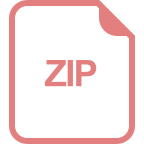
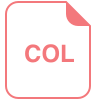
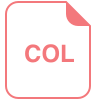
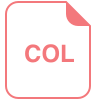
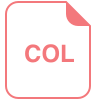
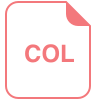
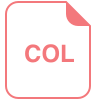